Development Environments: C++ Programming Setup
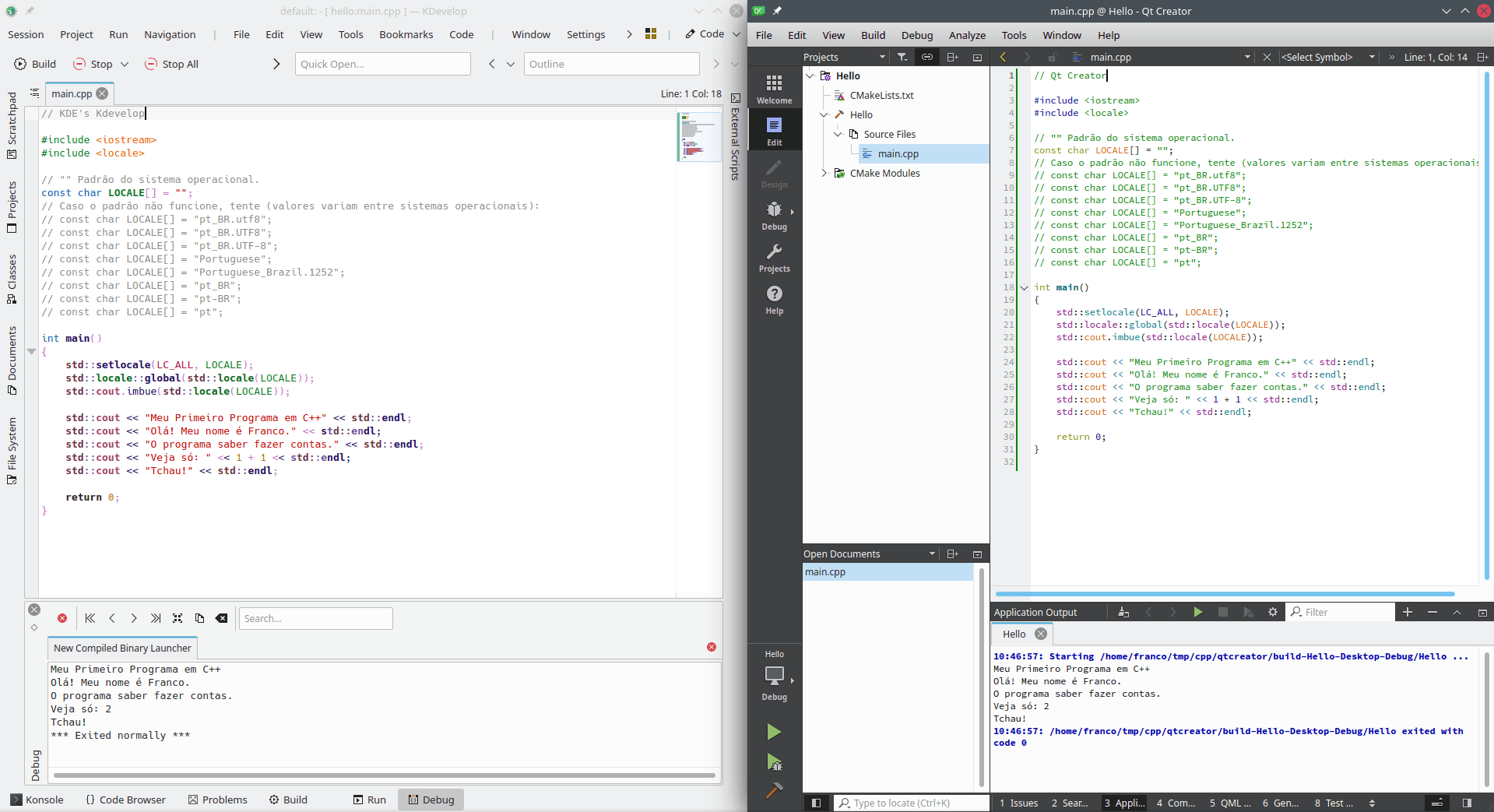
Image credits: Image created by the author using the program Spectacle.
Requirements
The development environment configuration to start programming in C++ is virtually the same used for the C programming language. Thus, instead of repeating the contents, you can find instruction on this link describing how to configure a development environment for C. The simplest option for beginners still is using an IDE. A good option is Code::Blocks.
After installing the compiler or an Integrated Development Environment (IDE), return to this page for your first tests with C++.
One of the main differences is that there exists Linux distributions that requires installing the g++
compiler instead (or besides) gcc
.
On others, gcc
includes g++
.
In reality, g++
is part of gcc
.
gcc
is an acronym for GNU Compiler Collection.
The collection includes compilers for several languages; for instance, for C, C++, Objective-C, D, Fortran, Ada, and Go.
Thus, is it possible to compile C++ code in some operating systems using gcc
.
In some cases, a .cpp
extension instead of a .c
can suffice.
C++ as the First Programming Language
Python and Lua lead my list of recommended programming languages for beginners, as previously commented in introduction about development environments; the third option is JavaScript, due to the Internet.The C programming language is far on my list of recommendations for beginners. C++ is as far as C (or even farther).
The reasoning is that C++ is more complex than C as a language, though it does offer a better standard library, with fewer surprises (and lower potential for errors). In particular, there are some features that selected operations on C++ easier than in C, such as a data type (as a class) to manipulate strings, collections for data structures, facilities to allocate and free memory, and operator overloading. However, the C++ language is more complex syntactically than C.
In fact, C++ grammar allows writing programs that are syntactically valid, though cannot be compiled (an example and another, more complex example). Thus, it can be suggested that C++ is an unnecessary difficult language to start learning programming.
Moreover, the previous considerations made for using C as a first programming language apply to C++.
In short, if you can choose, I recommend starting with Python, Lua, and JavaScript. It is better to focus on learning programming concepts, logic and computational thinking first. You can return to C++ once you are more experienced. It will be probably simpler and more productive to learn C++ once you understand the basics.
About C++
C++ shares many advantages and disadvantages of C, and add several others to both categories. Thus, the comments made in About C are also relevant for C++.
Like C, C++ is a compiled language, offering high performance and efficiency when it is used correctly. Nevertheless, C++ is harder to be used correctly than C, for it is a more complex language. While C is simple regarding its resources, C++ is a multi-paradigm programming language, which allows choosing different ways to program with the language. It is even possible that two people who program in C++ use radically different features from the language. As a result, each person can use resources and features that the other may not know.
Like C, C++ allows programming at low and medium levels (including the possibility of including assembly code). Unlike C, it also allows programming at higher levels (including using paradigms such as Object Orientation and Functional).
The language is a good choice for many use cases that C is also suitable: operating systems, compilers, interpreters, and systems and libraries requiring high performance. On the other hand, C++ is not always suitable for embedded systems (although there are guidelines (MISRA) for using the language).
In particular, C++ is the language of choice for many game engines of digital and video games. There are games that use C++ in combination with a scripting language. Parts requiring more efficiency are commonly written in C++. Parts that allow less efficiency can be written in other languages, such as Lua.
For advanced level programming, a significant difference between C and C++ is the machine code generated by the compiler. While code generated by C can be close to its source code, the code generated by C++ can be very different (it depends on what resources were used for programming).
For C++ documentation, you can use the following resources:
- Language standards;
- GNU C++ Library (libstdc++) documentation. In some Linux distributions, it is possible to use manual pages (as in C):
man std::stringstd::basic_string< _CharT, _Traits, _Alloc >(Library Functions Manstd::basic_string< _CharT, _Traits, _Alloc >(3) NAME std::basic_string< _CharT, _Traits, _Alloc > - Managing sequences of characters and character-like objects. SYNOPSIS
- The GNU C Library (glibc) documentation;
- MSDN, for Microsoft's `MSVC` compiler;
- cppreference.com;
- cplusplus.com.
Versions
C++ has had an even greater resurgence on the last years than C, including a modernization for the resources, features and libraries provided by the language.
The initial standardization of the occurred in 1998, with revisions in 2003 (C++03). The C++ committee was aiming for a new standard before the end of the 2010s; however, it was concluded in 2011 (C++11).
The C++11 standard has significantly modernized the language, adding features present in other higher level languages, such as Python. The evolution keeps accelerated, with the new standards C++14, C++17 and C++20, the current one. This next standard is expected in 2023 (C++23).
Nevertheless, it is important noticing that each compiler has its own rhythm to provide new features. For a comparative table, you can consult cppreference.com .
Furthermore, there are compilers that require using specific flags (values that represent a state or an occurrence of a condition) to enable modern features. Thus, if a compilation fails when using a compiler that should have provided a feature, you should check whether it is necessary to use a flag to enable it.
Additional Remarks
All information provided for the C programming language in Compilers and Programs apply to C.
Cross-compilation, link (and linker), header and library directories, and build systems operate the same way.
If you use g++
, it is often enough to swap the term gcc
by g++
, and C source code by C++ source code; the parameters should be the same.
Therefore, C++ does not simplify C's compiling process. In reality, some C++ compilers make the process more complex, due to Application Binary Interface (ABI) and name mangling.
There are compilers (particularly MSVC
circa 2014) that generate incompatible binaries between different versions of the same compiler for a very same source code.
Whenever that happens, it is necessary to recompile all libraries and dependencies with the exact version of the compiler that will be used in the project.
Fortunately, MSVC
situation
Compiler and Text Editor
To install a compiler, access Compiler and Text Editor in the page describing development environments for C.
Linux
Quick access to how to install a compiler on Linux.
The setup is similar; it is enough following the indications to install g++
on Linux distributions on which gcc
does not include the C++ compiler by default.
macOS
Quick access to how to install a compiler on macOS.
Windows
Quick access to how to install a compiler on Windows.
Environment Test
You can start to program in C++ after installing the compiler (or an IDE with a compiler).
To use the compiler, run its binary file or type g++
(the name can vary on Windows, depending on the installation; for instance, it can be named mingw
) in a command line interpreter (this requires setting up the PATH
for manual installs; click here for information on how to configure it).
This section presents the same examples used in JavaScript, Python and Lua for purposes of testing and comparing the languages.
To start, you can create a file with the following contents (std::cout
is an object that allows writing text to the console -- documentation; std::endl
inserts a line break -- documentation):
#include <iostream>
int main()
{
std::cout << "Olá, meu nome é Franco!" << std::endl;
// If you do not want to use std::endl, you can use:
// std::cout << "Olá, meu nome é Franco!" << '\n';
// Or yet:
// std::cout << "Olá, meu nome é Franco!\n";
// `\n` instead of using std::endl can result in better
// performance for your program.
return 0;
}
The message means "Hello, my name is Franco!" in Portuguese. Instead of translating it to English, it uses accented characters for further explanations.
Choose a name for the file (for instance, ola.cpp
) and compile it:
g++ ola.cpp -o ola.exe
The result will be a program called ola.exe
as an executable file.
For a better integration with your platform, choose (or omit) a file extension according to your operating system:
Linux:
g++ ola.cpp -o ola
Windows (choose between
gcc
ormingw
, according to your setup):g++ ola.cpp -o ola.exe
macOS:
g++ ola.cpp -o ola
To run your program:
Linux:
./olaOlá! Meu nome é Franco.
Windows:
# For use with `cmd.exe`: ola.exeOlá! Meu nome é Franco.
If you are using
MSYS2
onbash
instead ofcmd
, the process is similar to Linux; that means you should run the program as./ola.exe
:# For use with `MSYS2`: ./ola.exeOlá! Meu nome é Franco.
macOS:
./olaOlá! Meu nome é Franco.
Error: Stray Character in Program
This is the same problem explained for the C language. To solve it, follow the same steps.
Where Are the Accents?
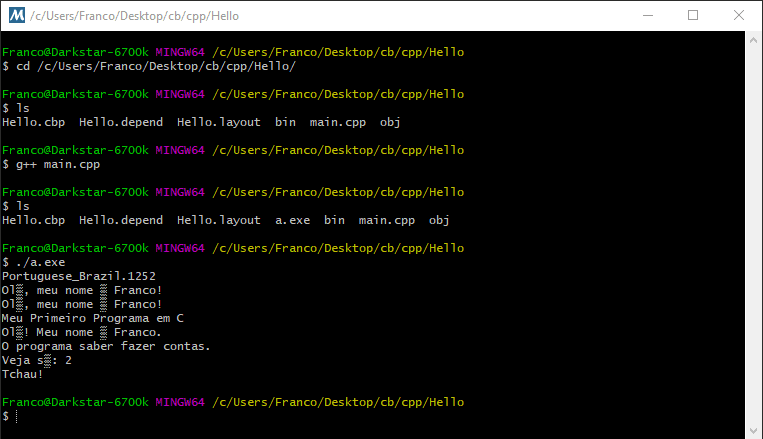
Once again, it is the sample problem explained for the C language. The explanation is the same (the locale should be configured), though the solution depends on operating system and compiler.
As in C, the correct value for the locale can vary.
Thus, you should pick a single line from the next examples to declare LOCALE
.
For instance, const char LOCALE[] = "Portuguese_Brazil.1252";
may work on Windows.
On the other hand, const char LOCALE[] = "pt_BR.UTF-8";
should work on Linux.
If you operating system's language is Portuguese, the simplest option is keeping the standard option: const char LOCALE[] = "";
.
An empty value (""
) uses the default locale value set for the operating system.
If you are reading this page in English, it is likely that your operating system's language is not Portuguese.
If you want to see the accented characters, you will need to use one the non-empty (that is, different from ""
) previous values.
In C++, the locale configuration requires using:
std::setlocale
(documentation);std::locale
(documentation);std::cout.imbue()
(documentation);
The next step depends on the operating system. If you use Linux, the solution is similar to C.
#include <iostream>
#include <locale>
// "" Standard locale, as defined by the operating system.
const char LOCALE[] = "";
// If the default does not work, you can try (values vary according to operating system):
// const char LOCALE[] = "pt_BR.utf8";
// const char LOCALE[] = "pt_BR.UTF8";
// const char LOCALE[] = "pt_BR.UTF-8";
// const char LOCALE[] = "Portuguese";
// const char LOCALE[] = "Portuguese_Brazil.1252";
// const char LOCALE[] = "pt_BR";
// const char LOCALE[] = "pt-BR";
// const char LOCALE[] = "pt";
int main()
{
std::setlocale(LC_ALL, LOCALE);
std::locale::global(std::locale(LOCALE));
std::cout.imbue(std::locale(LOCALE));
// Next, you can write the desired text.
std::cout << "Olá, meu nome é Franco!" << std::endl;
return 0;
}
If you use Windows, the scenario is more complicated.
The support for UTF-8 on Windows is a work in progress. There is an option to configure the whole operating system called Beta: Use Unicode UTF-8 for worldwide language support. However, as it is meant for testing, it can be unstable.
Thus, the alternative depends on compiler support to decode Portuguese_Brazil.1252
.
In Visual Studio 2019
with MSVC
, the solution is similar to Linux:
#include <iostream>
#include <locale>
// "" Standard locale, as defined by the operating system.
const char LOCALE[] = "";
// If the default does not work, you can try (values vary according to operating system):
// const char LOCALE[] = "pt_BR.utf8";
// const char LOCALE[] = "pt_BR.UTF8";
// const char LOCALE[] = "pt_BR.UTF-8";
// const char LOCALE[] = "Portuguese";
// const char LOCALE[] = "Portuguese_Brazil.1252";
// const char LOCALE[] = "pt_BR";
// const char LOCALE[] = "pt-BR";
// const char LOCALE[] = "pt";
int main()
{
std::setlocale(LC_ALL, LOCALE);
std::locale locale = std::locale(LOCALE);
std::locale::global(locale);
std::cout.imbue(locale);
std::printf("Olá, meu nome é Franco!\n");
std::cout << "Olá, meu nome é Franco!" << std::endl;
return 0;
}
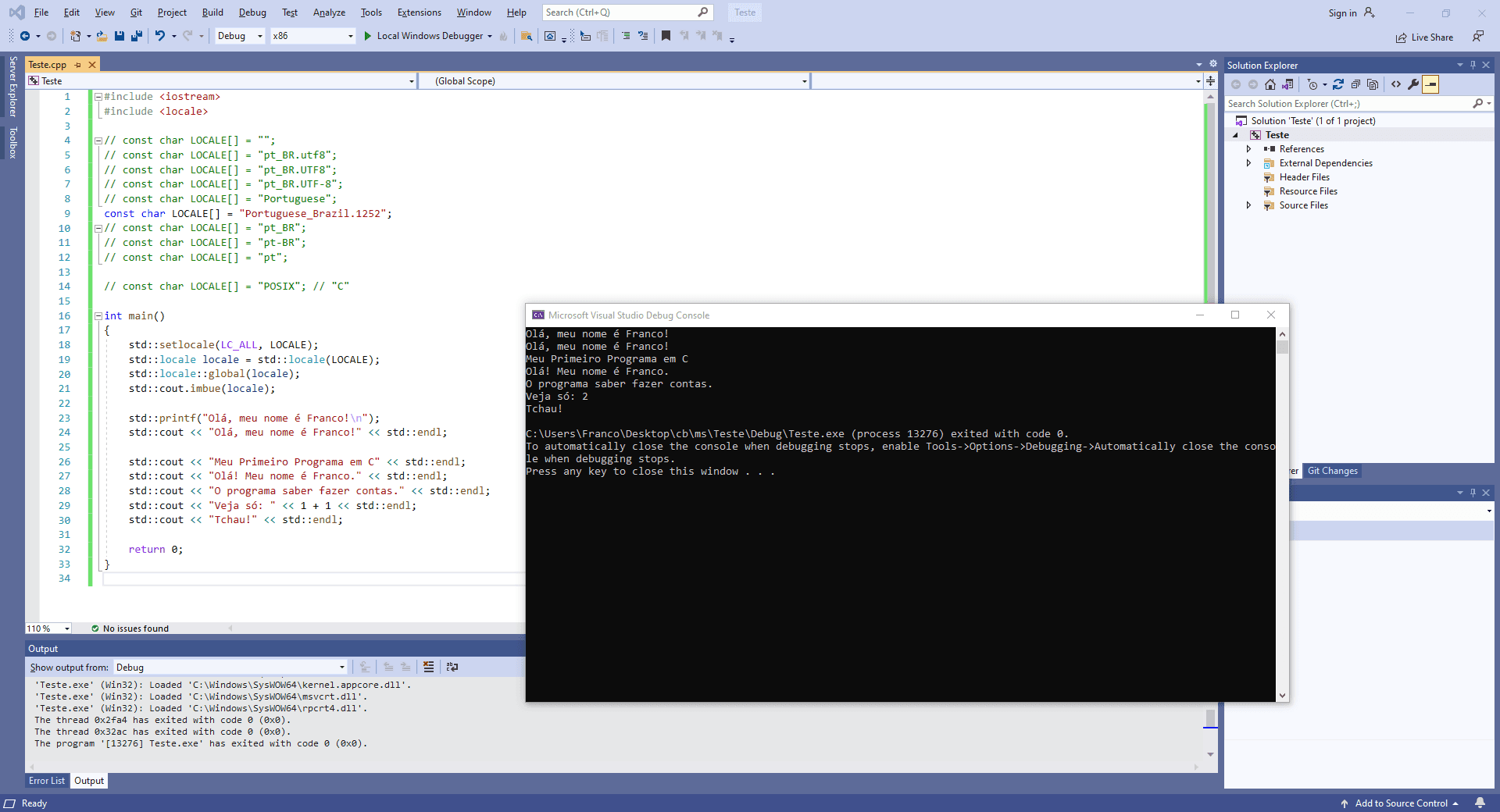
However, mingw
(gcc
/ g++
) only accepts the values ""
, "C"
and "POSIX"
for locale on Windows.
Although it is possible to retrieve the correct locale value (for instance, Portuguese_Brazil.1252
), it does not seem to be possible to set that value to configure std::locale::global()
e std::cout.imbue()
.
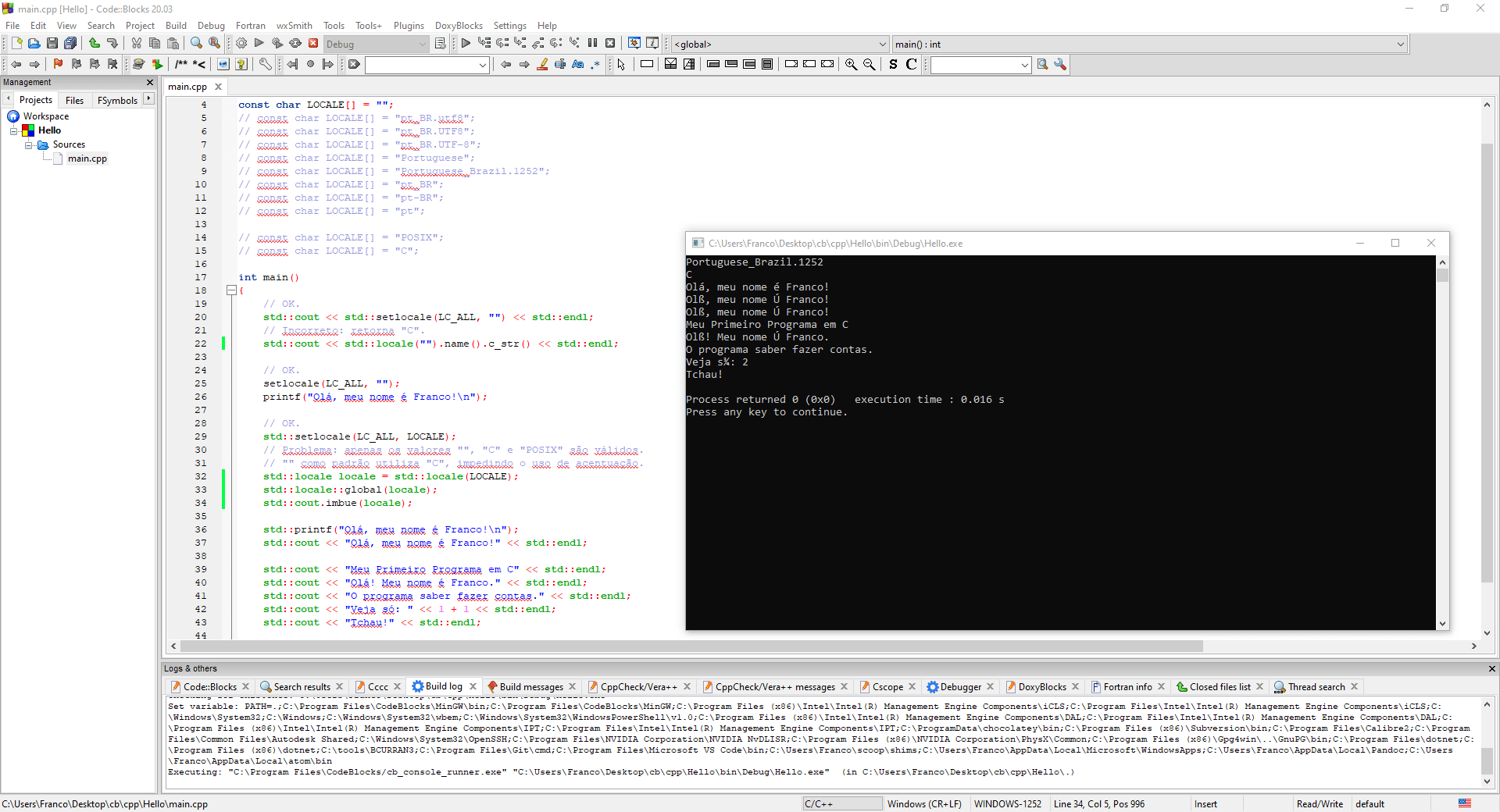
setlocale()
from C works correctly for C++, if you wish to use printf()
.
On the other hand, using std::cout
with accents may not work.
If you set only std::setlocale()
, accents may work, though it would be more correct to perform the other two settings as well.
#include <iostream>
#include <locale>
// "" Standard locale, as defined by the operating system.
const char LOCALE[] = "";
// If the default does not work, you can try (values vary according to operating system):
// const char LOCALE[] = "pt_BR.utf8";
// const char LOCALE[] = "pt_BR.UTF8";
// const char LOCALE[] = "pt_BR.UTF-8";
// const char LOCALE[] = "Portuguese";
// const char LOCALE[] = "Portuguese_Brazil.1252";
// const char LOCALE[] = "pt_BR";
// const char LOCALE[] = "pt-BR";
// const char LOCALE[] = "pt";
// Besides "", only the following values are valid for std::locale::global() and
// std::cout.imbue() on g++ for Windows:
// const char LOCALE[] = "POSIX";
// const char LOCALE[] = "C";
int main()
{
// OK.
std::cout << std::setlocale(LC_ALL, "") << std::endl;
// Incorreto: returns "C".
std::cout << std::locale("").name().c_str() << std::endl;
// OK.
setlocale(LC_ALL, "");
printf("Olá, meu nome é Franco!\n");
std::cout << std::locale("").name() << '\n';
// OK.
std::setlocale(LC_ALL, LOCALE);
// Problem: only the values "", "C" and "POSIX" are valid.
// "" as the default uses "C", hindering the use of accents.
// std::locale locale = std::locale(LOCALE);
// std::locale::global(locale);
// std::cout.imbue(locale);
std::printf("Olá, meu nome é Franco!\n");
std::cout << "Olá, meu nome é Franco!" << std::endl;
return 0;
}
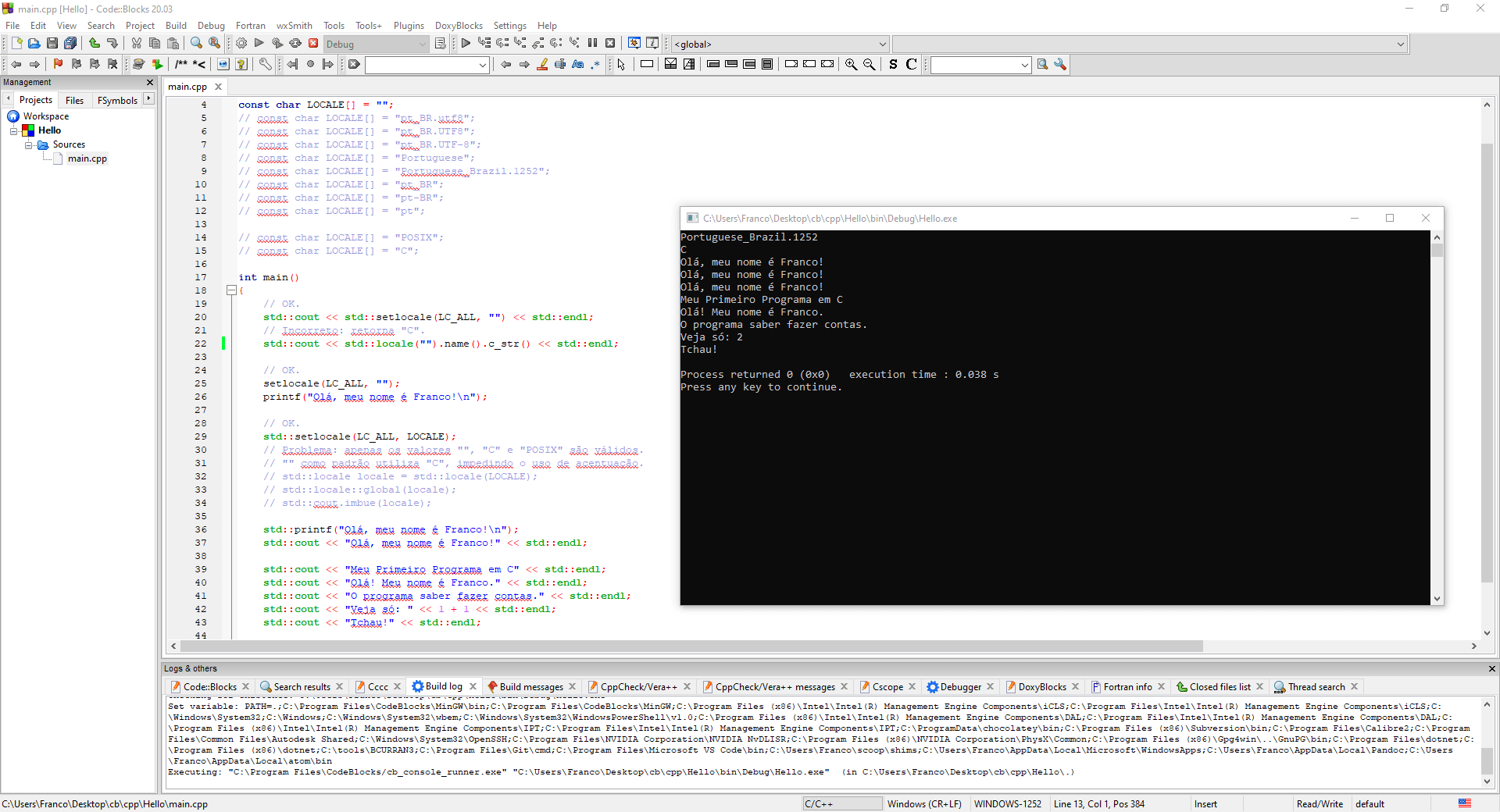
There are alternatives such as using the library International Components for Unicode (ICU). However, external libraries add extra steps that are unnecessary for beginners.
In the future, perhaps that the values from MSVC
work on mingw
.
Nevertheless, at this time, it is simpler to live with incorrect characters (or not work write them) if accents do not work with std::cout
instead of using printf()
in C++ for learning activities.
For beginners, it is simpler to use std::cout
in C++ than printf()
, because std::cout
does not require parameters for conversion nor using other specific methods to print (for instance, for strings).
Back to the Tests
After the issue of accents, you can create a second C++ source code file to continue the tradition from previous languages.
As it was the case for C, this will be your first program, though the suggested name will be first-program.cpp
.
You can change it, provided that you also modify the name in the compile line.
If the filename contains spaces, write the parameter to the compiler between double quotes (for instance, as gcc "Name with Spaces.cpp" -o "My Program.exe"
).
#include <iostream>
int main()
{
std::cout << "My First C++ Program" << std::endl;
std::cout << "Hello! My name is Franco." << std::endl;
std::cout << "The program can do Math." << std::endl;
std::cout << "Check it out: " << 1 + 1 << std::endl;
std::cout << "Bye!" << std::endl;
return 0;
}
To compile and run your program:
Linux:
g++ first-program.cpp -o first-program./first-programMy First C++ Program Hello! My name is Franco. The program can do Math. Check it out: 2 Bye!
Windows (if you are using
MSYS2
withbash
instead ofcmd
, the process is similar to Linux; that means you should run the program as./first-program.exe
):g++ first-program.cpp -o first-program.exefirst-program.exeMy First C++ Program Hello! My name is Franco. The program can do Math. Check it out: 2 Bye!
macOS:
g++ first-program.cpp -o first-program./first-programMy First C++ Program Hello! My name is Franco. The program can do Math. Check it out: 2 Bye!
Congratulations! If you have gotten to this point, now you understand how an IDE compiles and run programs behind the scenes. The next section shows how IDEs make the process easier, especially for beginners.
IDEs
The recommendations for C++ IDEs are the very same proposed for C. Instructions about how to use them are also identical.
Thus, to avoid unnecessary repetitions, instructions and comments are available in the page discussing how to set up a development environment for C.
Code::Blocks
Quick access: Code::Blocks.
Dev-C++
Quick access: Dev-C++.
KDevelop
Quick access: KDevelop.
Qt Creator
Quick access: Qt Creator.
Visual C++ / Visual Studio
Quick access: Visual Studio.
Xcode
Quick access: Xcode.
Other Options
There are many other options of IDEs for C and C++, including:
First Steps to Begin Programming in C++
The following source code snippets illustrate some resources of the C++ programming language. At this time, it is not necessary to fully understand them; the purpose is showing resources and the syntax of the language.
There are two ways of using the code. You can:
- Write and run the code in the IDE of your choice;
- Write the code using a text editor, generate a program using the file with code in a compiler, and run the generated program in your computer.
This simplest and fastest way is to use an IDE.
The examples are the same adopted for JavaScript. Therefore, you can compare languages, and notice similarities and differences between them.
Getting to Know C++ Through Experimentation
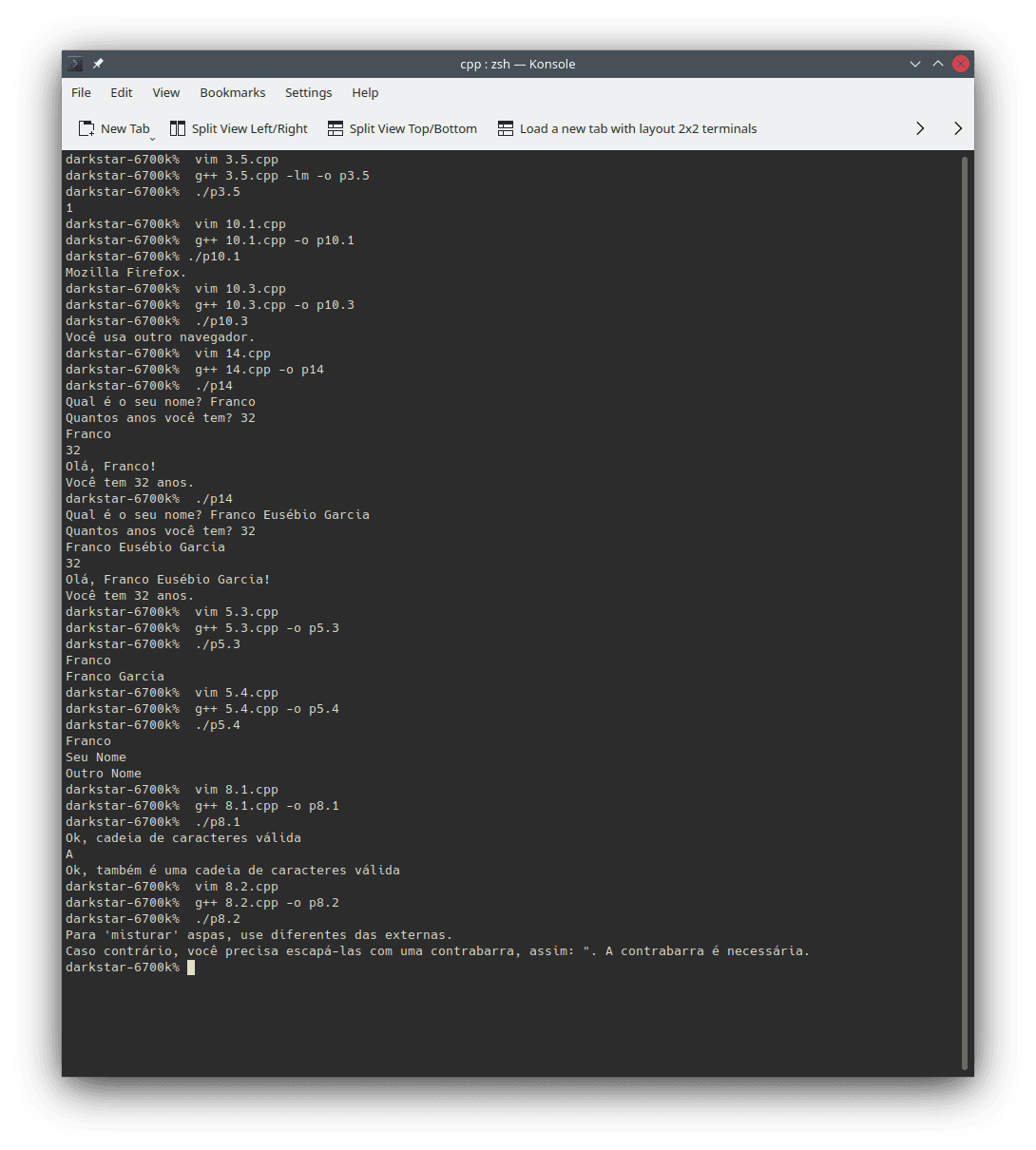
Text writing:
// g++ 1.cpp -o p1 #include <iostream> int main() { std::cout << "One line." << std::endl; std::cout << "Another line." << std::endl; std::cout << "One line.\nAnother line." << std::endl; return 0; }
To compile and run without an IDE, create a file named
1.cpp
with the previous contents and type:g++ 1.cpp -o p1 ./p1
You can choose other name for the source code file (
.cpp
) and for the executable to be compiled. In the next examples, the compilation line will be commented in the first line of the source code.If you do not want to create a file for each example, you can erase the contents and write the code from the next example. If you do want to save a copy of each example, (at this moment) it is necessary to create individual files.
Comments (text that is ignored by the interpreter; documentation):
// g++ 2.1.cpp -o p2.1 #include <iostream> int main() { std::cout << "Compiler processes" << std::endl; // Compiler ignores. return 0; }
// g++ 2.2.cpp -o p2.2 int main() { /* <- Here the comment starts. It can spawn multiple lines. Here it ends -> */ return 0; }
// g++ 2.3.cpp -o p2.3 int main() { /* It can also start and end in the same line. */ return 0; }
// g++ 2.4.cpp -o p2.4 int main() { // Though, for single line comments, it is easier to use this comment style. return 0; }
Mathematics operations:
Sum, subtraction, multiplication and division (documentation):
In C++, the division of two integer numbers result in an integer number (the remainder is truncated). To perform a real number division, at least one of the numbers must be real. For instance, compare the values of the divisions
6 / 4
and6 / 4.0
.
// g++ 3.1.cpp -o p3.1 #include <iostream> int main() { std::cout << 1 + 1 << std::endl; std::cout << 2 - 2 << std::endl; std::cout << 3 * 3 << std::endl; std::cout << 4 / 4.0 << std::endl; // What does happen if you try to divide by 0? Try it! return 0; }
- Math expressions:
// g++ 3.2.cpp -o p3.2 #include <iostream> int main() { std::cout << 1 + 2 - 3 + (4 * 5) / 6.0 << std::endl; return 0; }
- Power (documentation: pow()):
// g++ 3.3.cpp -lm -o p3.3 #include <iostream> #include <cmath> int main() { std::cout << std::pow(5, 2) << std::endl; return 0; }
- Square root (documentation: sqrt()):
// g++ 3.4.cpp -lm -o p3.4 #include <iostream> #include <cmath> int main() { std::cout << std::sqrt(25) << std::endl; return 0; }
// g++ 3.5.cpp -lm -o p3.5 #include <iostream> // In some compilers, you may have to remove the comment from the next line // to use MATH_PI. // #define _USE_MATH_DEFINES #include <cmath> int main() { std::cout << std::sin(M_PI / 2.0) << std::endl; // Seno. return 0; }
Comparisons (besides
false
andtrue
,0
meansfalse
and non-zero meanstrue
):- Equality (documentation; string):
// g++ 4.1.cpp -o p4.1 #include <iostream> int main() { std::cout << (1 == 2) << std::endl; // Equal: both equals are required! std::cout << (1 != 2) << std::endl; // Different. return 0; }
// g++ 4.2.cpp -o p4.2 #include <iostream> #include <string> int main() { std::cout << ("Franco" == "Franco") << std::endl; std::cout << ("Franco" != "Franco") << std::endl; std::cout << ("Franco" != "Seu Nome") << std::endl; // C++ differs lower case characters from upper case ones (and vice-versa). std::cout << ('F' == 'f') << std::endl; // Single characters in C++ are integer numbers. std::cout << ('F' != 'f') << std::endl; std::cout << ("Franco" == "franco") << std::endl; std::cout << ("Franco" != "franco") << std::endl; return 0; }
- Other comparisons (documentation):
// g++ 4.3.cpp -o p4.3 #include <iostream> int main() { std::cout << (1 < 2) << std::endl; // Less than. std::cout << (1 > 2) << std::endl; // Greater than. std::cout << (1 <= 2) << std::endl; // Less or equal than. std::cout << (1 >= 2) << std::endl; // Greater or equal than. return 0; }
Variables and assignment (documentation):
Variables are like boxes that store values put inside them. The assignment operator (a single equal sign (
=
) in C++) commits the storage.// g++ 5.1.cpp -o p5.1 #include <iostream> int main() { int x = 123; std::cout << x << std::endl; return 0; }
// g++ 5.2.cpp -o p5.2 #include <iostream> int main() { float result = 123.456 + 987.654; std::cout << result << std::endl; return 0; }
You should note that you can declare a variable with a chosen name only once. However, you can change its value as many times as you need.
// g++ 5.3.cpp -o p5.3 #include <iostream> #include <string> int main() { std::string name = "Franco"; std::cout << name << std::endl; name = "Franco Garcia"; std::cout << name << std::endl; return 0; }
// g++ 5.4.cpp -o p5.4 #include <iostream> #include <string> int main() { std::string variables_can_vary = "Franco"; std::cout << variables_can_vary << std::endl; variables_can_vary = "Seu Nome"; std::cout << variables_can_vary << std::endl; variables_can_vary = "Outro Nome"; std::cout << variables_can_vary << std::endl; return 0; }
// g++ 5.5.cpp -o p5.5 #include <iostream> int main() { bool logical_value = true; std::cout << logical_value << std::endl; logical_value = false; std::cout << logical_value << std::endl; logical_value = (1 + 1 == 2); return 0; }
Constants (documentation):
// g++ 6.1.cpp -o p6.1 #include <iostream> const float PI = 3.14159; int main() { std::cout << PI << std::endl; return 0; }
// g++ 6.2.cpp -o p6.2 #include <iostream> const float E = 2.71828; int main() { std::cout << E << std::endl; E = 0; // Error; the value of a constant cannot be changed once set. return 0; }
Errors:
// g++ 7.cpp -o p7 #include <iostream> int main() { std::cout << Ooops!; // Text should be between double quotes. std::cou << "Incorrect name for cout" << std::endl; std::cout << 1 / 0 << std::endl; std::cout << 0 / 0 << std::endl; return 0; }
Strings for words and text (documentation):
// g++ 8.1.cpp -o p8.1 #include <iostream> int main() { std::cout << "Ok, this is a valid string\n" << std::endl; std::cout << 'A' << std::endl; // Single character. std::cout << "Ok, this " " is also a " " valid string" "\n"; return 0; }
// g++ 8.2.cpp -o p8.2 #include <iostream> int main() { std::cout << "If you want to 'mix' quotes, you have to use those different from the external ones.\n" << std::endl; std::cout << "Otherwise, you will need to escape them with a backslash, like this: \". The backslash is required.\n" << std::endl; return 0; }
Logical operations (documentation):
// g++ 9.cpp -o p9 #include <iostream> int main() { std::cout << (true && true) << std::endl; // This is a logical "and". std::cout << (true || true) << std::endl; // This is a logical "or". std::cout << (!false) << std::endl; // This is a logical "not". return 0; }
Conditions (documentation):
// g++ 10.1.cpp -o p10.1 #include <iostream> #include <string> int main() { std::string browser = "Firefox"; if (browser == "Firefox") { std::cout << "Mozilla Firefox." << std::endl; } return 0; }
// g++ 10.2.cpp -o p10.2 #include <iostream> #include <string> int main() { std::string my_browser = "Your Browser"; if (my_browser == "Firefox") { std::cout << "You use a browser from Mozilla." << std::endl; } else { std::cout << "You use another browser." << std::endl; } return 0; }
// g++ 10.3.cpp -o p10.3 #include <iostream> #include <cctype> #include <string> int main() { std::string i_use = "X"; int index = 0; for (char& character : i_use) { character = std::tolower(character); } if (i_use == "firefox") { std::cout << "You use a browser from Mozilla." << std::endl; } else if ((i_use == "chrome") || (i_use == "chromium")) { std::cout << "You use a browser from Google." << std::endl; } else if (i_use == "edge") { std::cout << "You use a browser from Microsoft." << std::endl; } else if (i_use == "safari") { std::cout << "You use a browser from Apple." << std::endl; } else if (i_use == "internet explorer") { std::cout << "You should use a more modern browser...\n" << std::endl; } else { std::cout << "You use another browser." << std::endl; } return 0; }
Loops (documentation: for, while, and do...while):
// g++ 11.1.cpp -o p11.1 #include <iostream> int main() { int i; for (i = 0; i < 5; i = i + 1) { std::cout << i << std::endl; } return 0; }
// g++ 11.2.cpp -o p11.2 #include <iostream> int main() { int j = 0; while (j < 5) { std::cout << j << std::endl; ++j; // The same as j = j + 1 and similar to j++ (there are slightly differences) } return 0; }
// g++ 11.3.cpp -o p11.3 #include <iostream> int main() { int k = 0; do { std::cout << k << std::endl; k++; } while (k < 5); return 0; }
Functions (documentation):
// g++ 12.cpp -o p12 #include <iostream> int my_function(int x, int y) { int result = x + y; return result; } int main() { // A function is a block of code that performs arbitrary processing as defined // by the programmer. // After the definition, you can execute the function whenever wanted, using // a function call. int z = my_function(12, -34); // This is an example of a function call. std::cout << z << std::endl; std::cout << my_function(1, 2) << std::endl; // This is another example. return 0; }
Data types (documentation):
// g++ 13.cpp -o p13 #include <iostream> #include <string> int main() { int integer_number = 1; int another_integer_number = -1; float real_number = 1.23; bool logic_value = true; // or false; it only can be either true or false. std::string string_for_text = "Text here. Line breaks use\nthat is, this will be at\n the third line.\n"; return 0; }
Input (documentation: std::getline e std::cin):
// g++ 14.cpp -o p14 #include <iostream> #include <string> int main() { // Once there is a request for a value, type one then press `enter`. std::cout << "What is your name? "; std::string your_name; std::getline(std::cin, your_name); std::cout << "How old are you? "; int your_age; std::cin >> your_age; std::cout << your_name << std::endl; std::cout << your_age << std::endl; std::cout << "Hello, " << your_name << "!" << std::endl; std::cout << "You are " << your_age << " years old." << std::endl; return 0; }
Congratulations! You Are Already Able to Write Any Program in C++
Well... Probably not. Like C, C++ is a complex language. In particular, C++ syntax can be hard to read and write when you are starting using the language. The syntax can become even harder to read when you start using specific C++ features such as templates.
However, the title of the section is technically correct.
Don't you believe it? You can find the explanation in the introduction to JavaScript
In short, it is not enough to learn the syntax of a language to solve problems using it. It is much more important learning programming logic and developing computational thinking skills than learning the syntax of a programming language. In fact, when you are in doubt about the syntax, you just have to consult the documentation. You do not have to memorize it.
After you learn a first programming language, it is relatively simple to learn other languages (that adopt similar programming paradigms to the first one). To verify this statement, you can open a new window in your browser and place it side by side with this one. Then, you can compare the source code blocks written in C++ (in this window) and in Python (in the second window).
If you wish to perform comparisons with other programming languages, the following options are available:
Next Steps
Once the development environment is configured, you can continue your system development journey.
To avoid repetitions, I recommend reading the page about configuring the JavaScript environment. Even if you are not interested at the language, many discussions are relevant to any programming language. Besides, you will understand a way to create Internet pages and will be able to create content for your browser.
Until GDScript (for Godot Engine), the topics describe develoment environment configurations for a few programming languages. After GDScript, the focus becomes basic concepts for learning programming.