Development Environments: Python Programming Setup
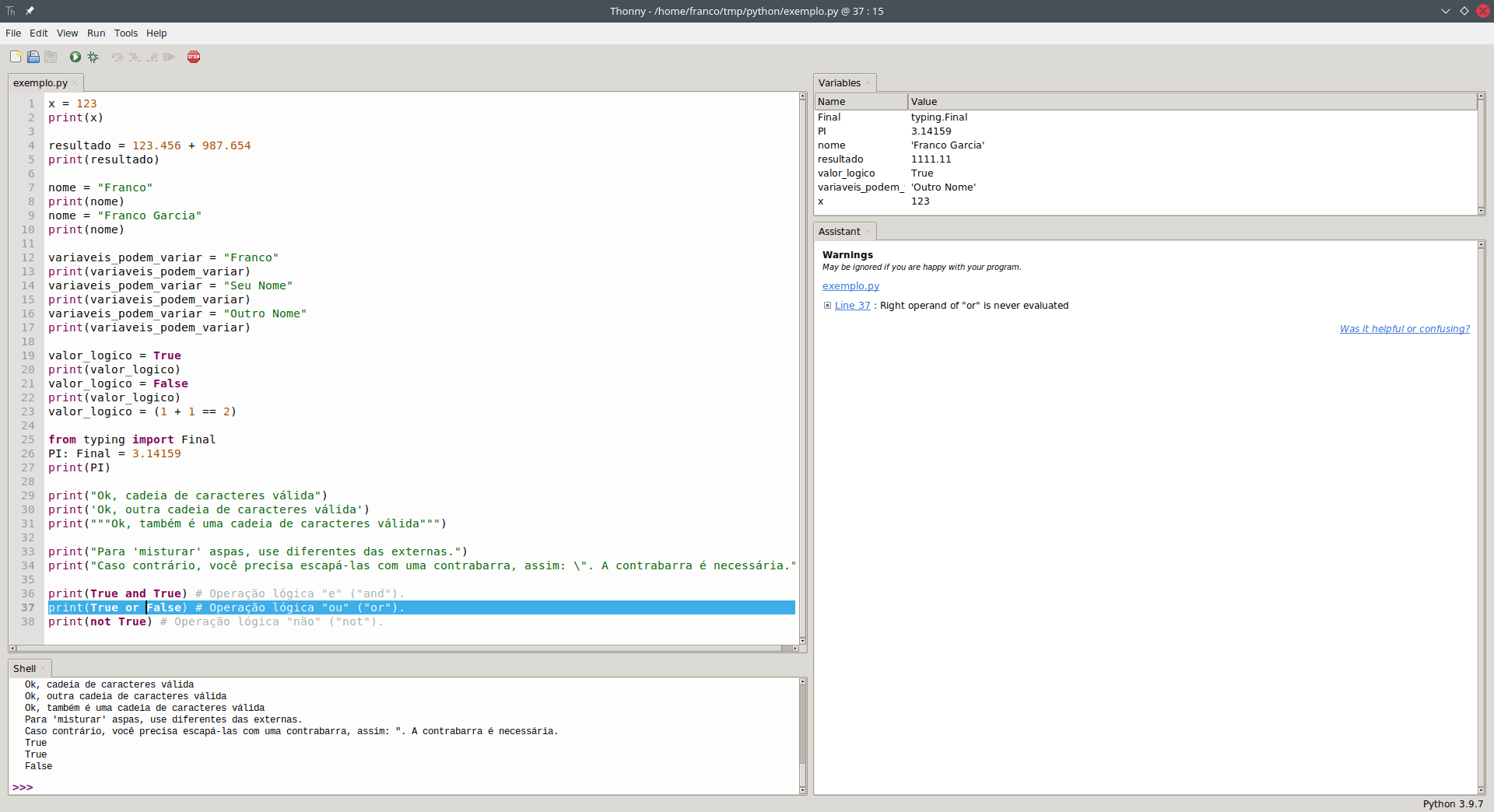
Image credits: Image created by the author using the program Spectacle.
Requirements
The requirements to start programming activities with Python are similar to those described for JavaScript: a text editor and an interpreter. However, beginners may find it more appropriate to start by using an Integrated Development Environment (IDE).
Similarly to most programming languages, there are two main options to start programming in Python:
- A text editor to write programs and the language's interpreter to run them;
- An IDE, which combines a text editor, the interpreter and other features into a single program.
It is also possible to use an online environment; for instance, I provide a Python code editor and interpreter for the first programming activities, which only requires your Internet browser. Nevertheless, sooner or later you will need to configure a programming environment in your computer.
If you opt to start by using an IDE (something I would suggest), my recommendation for beginners is Thonny (as it will be presented at an appropriate section).
If you have not yet installed an interpreter and a text editor, you can choose to install Thonny
instead as a single application to program with Python.
If you have already installed a Python interpreter, check if the IDLE IDE was installed together with it. For installs using Ninite or the installer from the official website, it is likely that IDLE has been installed and is ready to use without requiring further configuration.
If you choose the text editor route, you will need to install the Python interpreter.
Although optional, it may be desirable to add the interpreter's directory to your operating system's PATH
.
If you do now know how to install program, what is PATH
or how to configure it, you can refer the topic regarding how to install programs.
Alternatively, you can start with Thonny.
Video Version
This entry has a video version available on the YouTube channel. Although this text version is more complete, you can follow the video first, if you prefer it.
About Python
Python and Lua lead my list of recommended programming languages for beginners, as previously commented in introduction about development environments; the third option is JavaScript, due to the Internet.
The syntax of Python is concise and elegant, to the point of looking like the pseudocode for an algorithm. The simplicity and expressiveness are desirable and opportune features for beginners, for they can reduce chances of mistakes and the necessity of typing unnecessary code.
Notwithstanding the syntax, the language is one of the pioneers on popularizing the term batteries included, that refers to the extensive programming libraries provided by and for Python. The standard library covers many domains and has high quality. There are even more external libraries available for use, with applications for multiple domains of human knowledge and activities.
The trade-off is that the language's performance can be unsatisfactory when compared to some other languages. However, performance should be a main concern for beginners, whose main goal should be learning programming logic and computational thinking.
Performance is not a priority for all domains of computing or all problems for every domain. As a result, Python is a language with good acceptance both for the industry and the academy. Thus, there are opportunities to work with Python for both professional and academic scenarios.
Furthermore, Python is a good choice to prototype systems. The expressiveness of the language allows writing systems quickly to evaluate ideas and implementations of possible solutions. If the performance of prototype turns to be unacceptable, it is possible to implement a more mature version in languages such as C, C++, Rust or Go afterwards.
Versions
When this page was written, Python 3
was the main version of the language, although Python 2
was still used.
For learning and/or new projects, I would recommend using Python 3
.
Interpreter and Text Editor
It is important to note that root
or administrator
accounts should be used only to install the interpreter.
You should use it with a regular user account, with lower privileges.
Linux
In Linux distributions with package managers, it is almost immediate to install the Python interpreter. A few examples for popular distributions:
- Arch Linux:
pacman -S python
- Debian, Ubuntu or distributions based on the former ones:
apt-get install python3
- Fedora:
dnf install python3
- Gentoo: Python should be preinstalled.
macOS
On macOS environments, you can use brew
to install Python.
Windows
On Windows environments, the usual approach is downloading the interpreter from the official website.
You should select the most recent stable version (Lastest Python 3 Release), access the page e choose the installer (for instance, Windows installer (64-bit)
for 64-bit machines).
During the setup, it is convenient marking the option to add the program to the PATH
, to avoid having to set it yourself later.
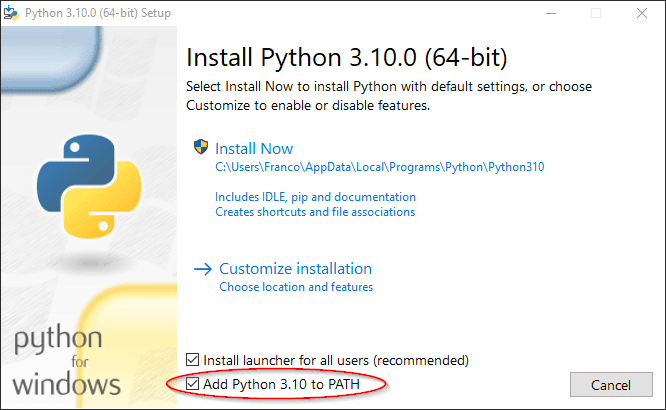
Alternatives to install the interpreter on Windows include:
- Ninite, Chocolatey or Scoop, as previously discussed, including `PATH` configuration;
- An IDE with an integrated interpreter (for instance, Thonny).
Environment Test
You can start to program in Python after installing the interpreter.
To do this, you start the interpreter (for instance, type python
followed by enter
in a command interpreter or search the operating system's main menu, such as the Start
menu on Windows, for python
and start the program).
pythonPython 3.9.7 (default, Aug 31 2021, 13:28:12)
[GCC 11.1.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
After starting the python
interpreter, the result will resemble the previous source code block.
You will type your code after the greater than symbols (>>>
).
For instance, you can write on the console with print()
(documentation).
To write a message, type something like print("Hello! My name is Franco.")
after the greater than symbols and press enter
.
The interpreter will write Hello! My name is Franco
and will wait your next command.
pythonPython 3.9.7 (default, Aug 31 2021, 13:28:12)
[GCC 11.1.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> print("Hello! My name is Franco.")Hello! My name is Franco.
>>>
From now on, you should follow the previous steps whenever you read a Python source code block in this page and wish to manually run in the interpreter.
To exit the interpreter, type quit()
followed by enter
or press Ctrl d
.
On Windows, the shortcut should be Ctrl z
followed by enter
.
The text in the images are from the Portuguese version of this page.
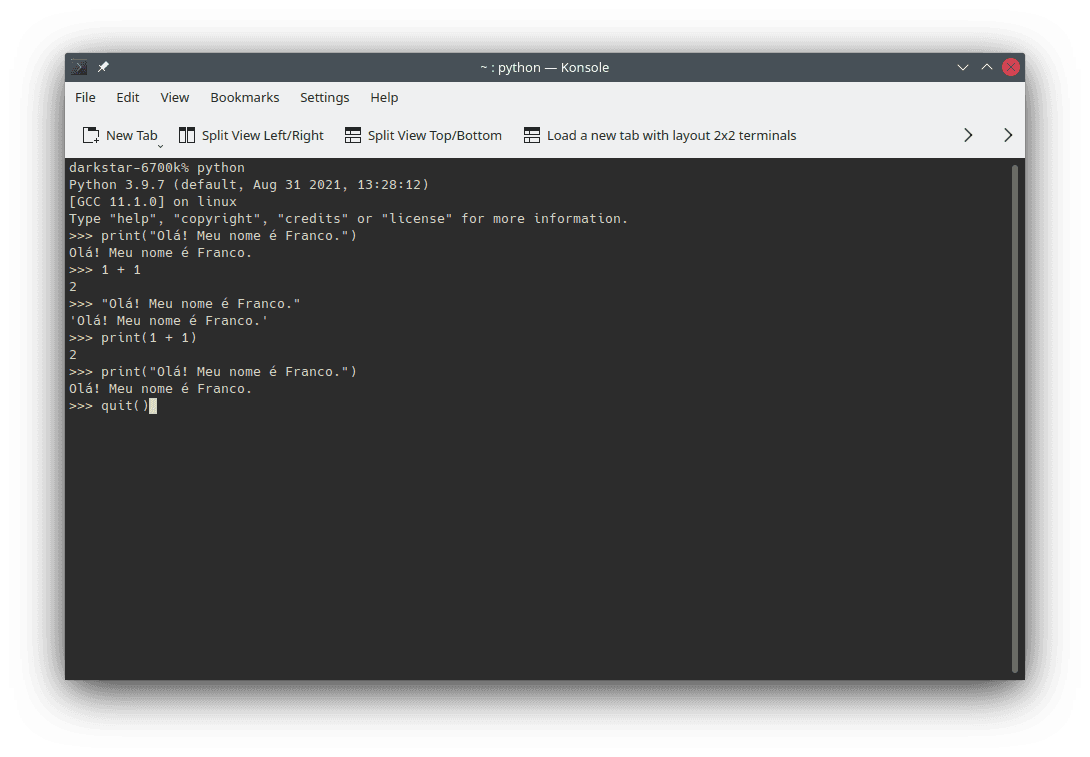
The interactive use of an interpreter outlined in the previous steps are called Read, Eval, Print, Loop
(REPL).
Coding in REPL system is an excellent way to learn programming, because you can test ideas and verify results (or errors) quickly.
Do you have an idea for a possible solution, though you are not sure whether it is correct?
Perhaps you have a "what if..." question?
You can test them using REPL.
If the solution is wrong, identify problems, make adjustments and run the code again.
You can repeat this process until the implementation is correct.
The process is fast, iterative and interactive.
REPL provides some additional conveniences.
Besides writing messages, you can perform mathematical operations directly in the interpreter.
In other words, you can use REPL as a replacement for calculators and spreadsheets.
For instance, type the following code and press enter
to find the result of the expression.
1 + 1
You can also type a string directly:
"Hello! My name is Franco."
However, you should note that those direct uses are only possible for interactive use, that is, in REPL style.
They are convenient ways to inspect values and variables, though they are not permitted in programs.
If you wish to perform the same operations in a source code file (a script), you should use print()
to write the results.
print(1 + 1)
print("Hello! My name is Franco.")
Thus, it can be convenient to create the habit of using print()
when you want to write in the console.
For instance, create a file called script.py
(you can choose any name that you want) with the following contents:
print("My First Python Script")
print("Hello! My name is Franco.")
print("The interpreter can do Math.")
print("Check it out: ", 1 + 1)
print("Bye!")
To run the script, open a command line interpreter (shell) in the directory that you have created the file and type python script.py
.
The Python interpreter will run the file provided as a parameter (the value script.py
after python
) and will show results from print()
calls.
python script.pyMy First Python Script
Hello! My name is Franco.
The interpreter can do Math.
Check it out: 2
Bye!
If the current directory of command line interpreter (shell) is not the directory of the file, you can change directory to the file's folder, or you can write the absolute path of the file.
Examples for absolute file paths can include C:\Users\Franco\Desktop\script.py
on Windows for a file in the desktop of a user account named Franco
or ~/Desktop/script.py
for a file in the desktop of a Linux machine.
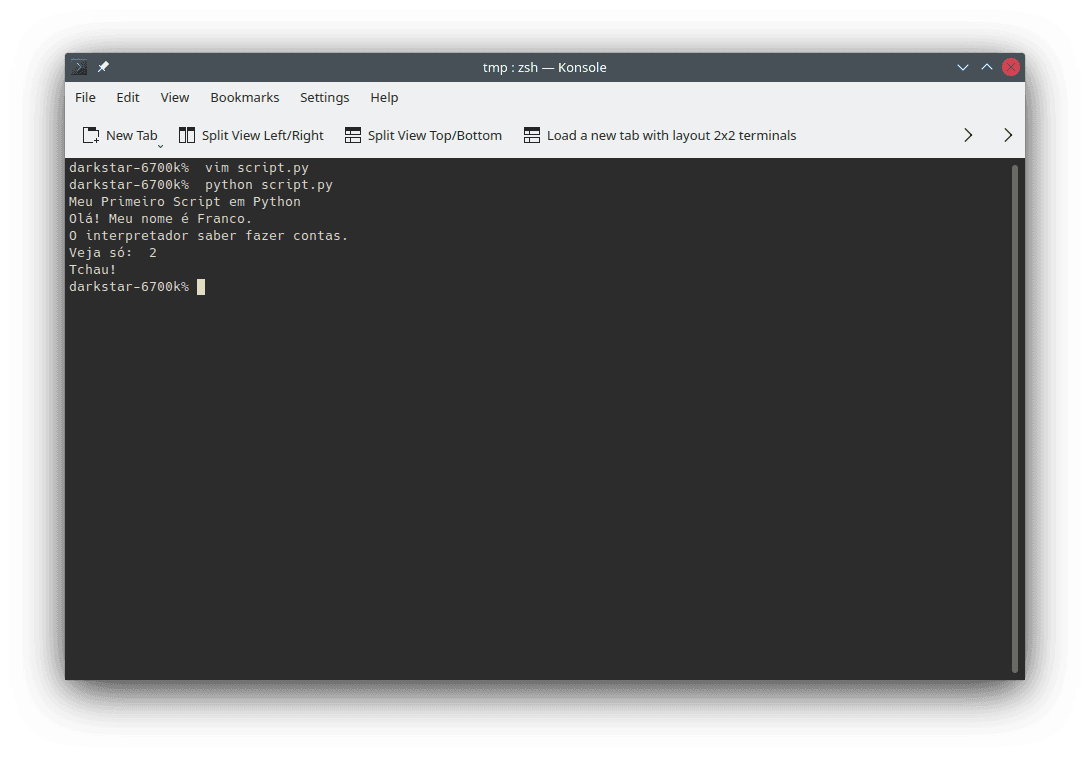
It is enough to know how to use the REPL and run a Python source code file for learning activities. In general, these two operations are also enough for most daily or professional uses of the programming language.
IDEs
To make programming activities easier for beginners, I would recommend using IDEs for most programming languages. Although Python, Lua and JavaScript can be considered exceptions due to the relative ease of obtaining and using an interpreter, IDEs still provide a more convenient learning experience.
In particular, many IDEs allow to run a file or project with a single click (normally on a triangle icon (🢒
) commonly used to play content eletroelectronics) or with a key press (usually F5
).
In other words, instead of starting a terminal session and typing the required commands to run your program, it is enough to press a button or a key.
Furthermore, there exists an IDE created specifically for beginners in the Python language called Thonny
.
Before proceeding, it is worthy noting that the usual features and shortcuts used by text editors should apply to most IDEs.
Thus, for instance, it should be possible to create new files using a shortcut such as Ctrl n
(or File / New
), as well as opening files with Ctrl o
(or File / Open
).
Thonny
Is Python your first even programming language?
University of Tartu (Estonia) has created an IDE for programming beginners called Thonny.
Thonny
setup includes an embedded Python interpreter; thus, it is enough to set up the IDE to start programming in the language, without further configuration.
The graphical user interface is simple and has few options, which is something that makes Thonny
different from most other IDEs (which are created for professionals, usually with user interfaces full of menus and icons).
For the very first uses it is even possible to restrict the IDE to a panel for writing source code and another panel to show the results of running the program.
As you learn and acquire more confidence, it is possible to add other panels, such as to display variables and for debugging.
Furthermore, Thonny
:
- Provides translations for some (human) languages, which can be chosen during the first use, or at any time in
Tools / Options / Language
(changing the settings in that screen requires restarting the IDE, that is, close it and open it again); - Requires low RAM (around 30--70 MB for simple programs) to run, making it a good option to older computers or with mode more hardware configurations, as well as a fast program in more recent machines;
- Provides resources to graphically illustrate program executions;
- Provides resources to learn some programming concepts (such as recursion);
- Provides visual indication for common beginners' syntax mistakes, such as forgetting to close quotes or parentheses;
- Provides visual indication for variables sharing a same name, but in different scopes;
- Has a graphical manager to install external libraries;
- Provides an option (available in
View / Assistant
) to explain some code errors (in English).
Thus, the IDE can be a good resource to assist many people learning programming.
Unfortunately, it does not support screen readers when this entry was written, making it inaccessible for people depending on the resource (for instance, blind people).
For those who depends on screen readers, Visual Studio Code
, PyDev
e PyCharm
can be more appropriate languages.
Lua can also possible be a better choice for a first programming language, because Python uses indentation to define scopes.
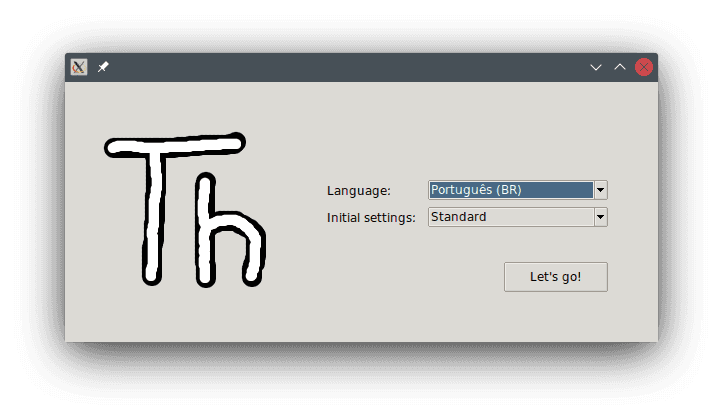
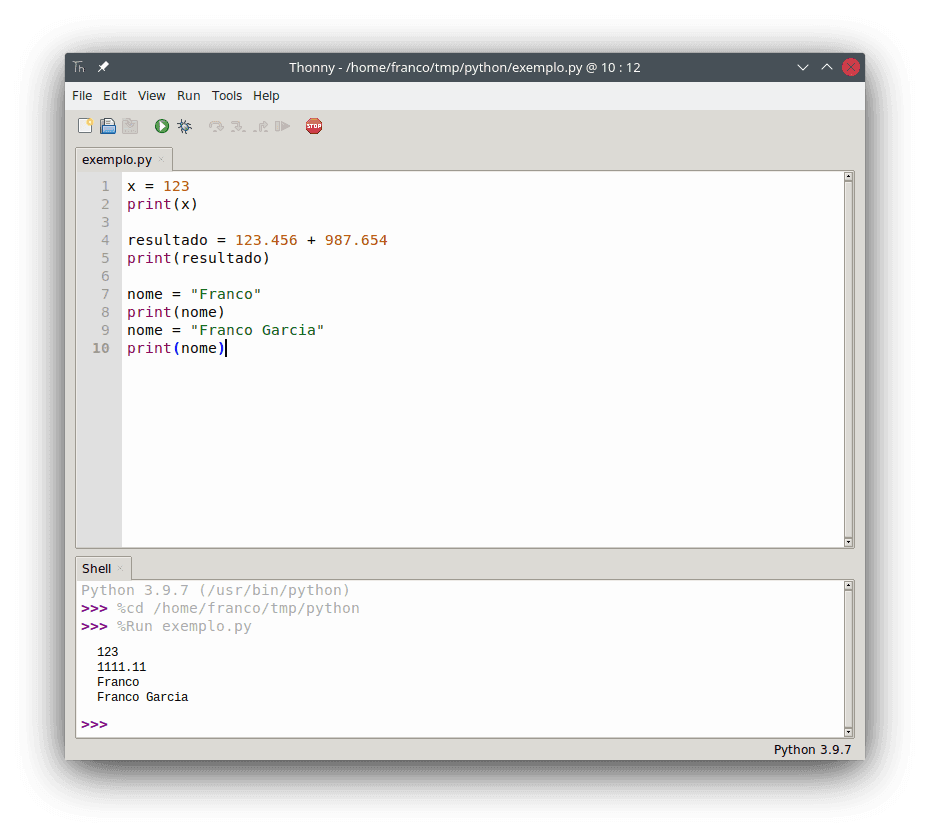
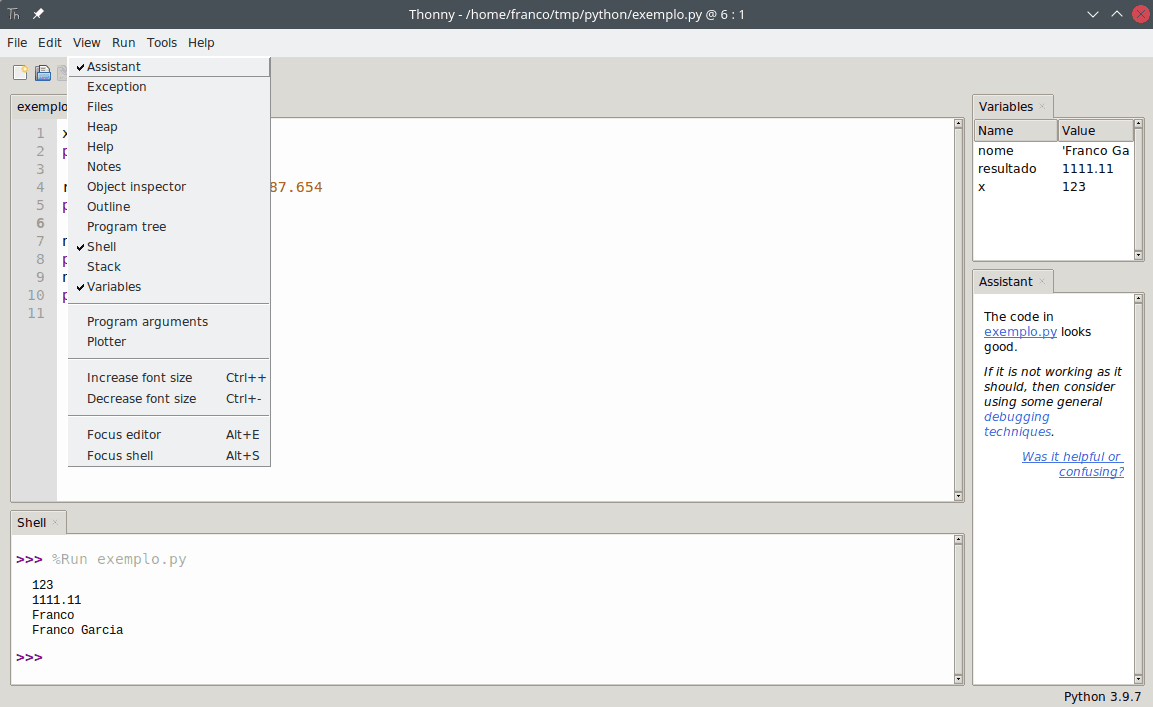
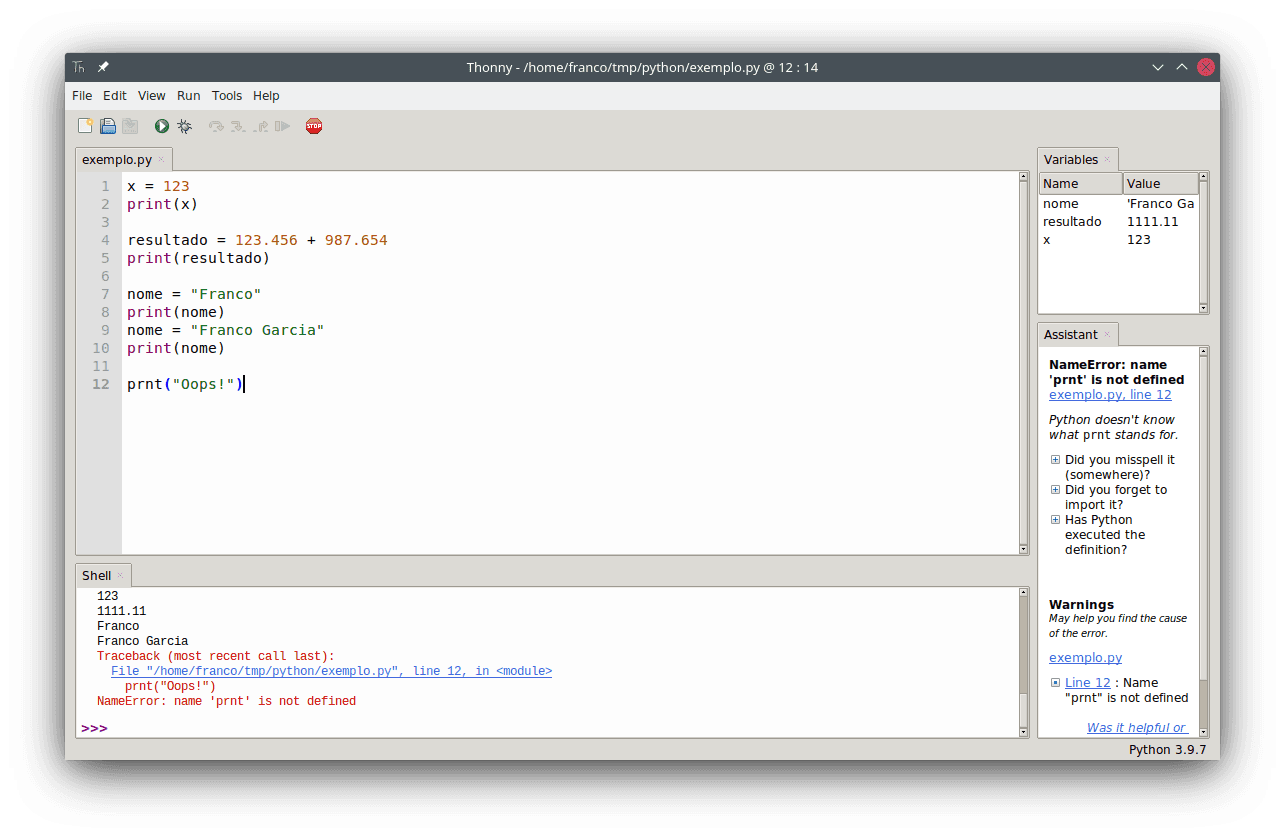
IDLE
Python's Integrated Development and Learning Environment (IDLE) is the Python's project official IDE. `IDLE` is also a simple IDE, aimed especially for beginners, with the advantage of being potentially installed automatically with the Python interpreter.In other words, depending on how you have set up the interpreter, you may have installed IDLE
at the same time.
If this is case, you can start IDLE to start programming.
For instance, Ninite installs IDLE together with Python.
To start using it on Windows, it is enough to search for the program name (IDLE
) in the Start menu and run it to start programming in Python.
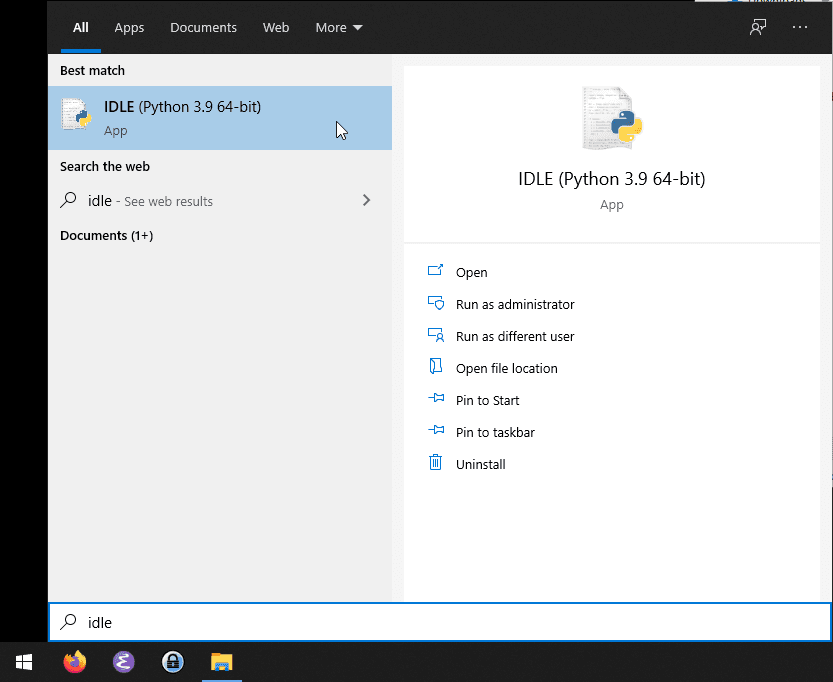
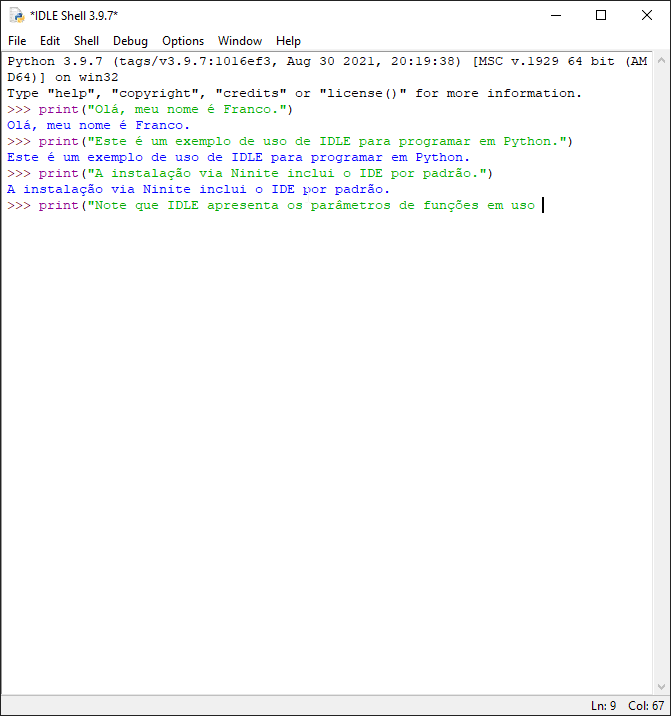
Jupyter Notebooks
For people who like taking notes while they study (and that want to keep the habit when learning programming), Jupyter (formerly Jupyter Notebook and IPython Notebook) can be an excellent option. It is an alternative to the excellent Org Mode Babel for Emacs, with the advantage of not requiring Emacs to those who do not use the text editor.
Jupyter
enables programmings in the form of an interactive text document, on which it is possible to mix code and explanations in a single artifact.
The practice can be an example of literate programming, on which text is created alongside the source code to explain how it works.
It is possible to test Jupyter
on the browser or install it locally in the machine.
To test it via browser, choose Try Classic Notebook or Try JupyterLab.
If you need to choose a program language, select Python.
For the new format (JupyterLab
), cells can be defined as code, text without formatting and Markdown formatted text.
The choice of type defines how a cell will be interpreted.
- To add a new cell, click the plus sign (
+
); - To choose the type of cell, choose an option in the dropdown menu;
- To run a cell, press the play button (the triangle). Results from previous cells can be referenced in the next ones. In other words, it is possible, for instance, to define a variable in a cell to access it in another, as well as load a library in a cell for future use;
- You can save the notebook's content locally in your machine using
File / Download
.
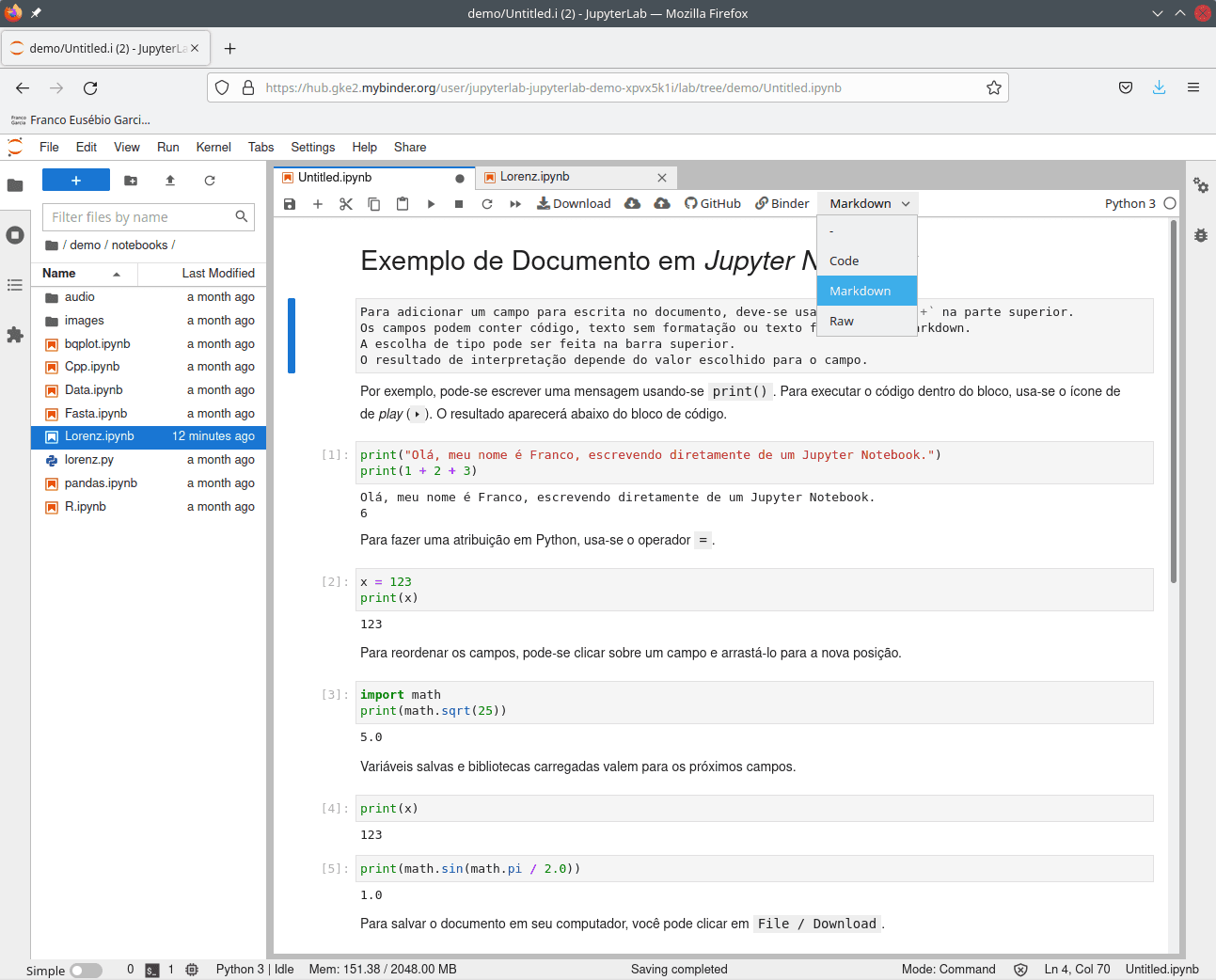
For an open source Jupyter project that is available in a public hosted repository (for instance, using GitLab or GitHub), it is possible to publish the notebook's contents online with Binder.
Other Options
Python support can be added to Visual Studio Code
with an extension.
In particular, the previous integration also supports Jupyter
.
Analogously, Python support can be added to Atom
with the extension IDE-python.
There are other IDE options, including:
- PyDev;
- Spyder;
- KDevelop;
- PyCharm (the Community Edition is free to use).
First Steps to Begin Programming in Python
The following source code snippets illustrate some resources of the Python programming language. At this time, it is not necessary to fully understand them; the purpose is showing resources and the syntax of the language.
There are three ways of using the code. You can:
- Type (or copy and paste) the code directly into the language's interpreter;
- Write and run the code in the IDE of your choice;
- Write the code using a text editor and run the created file in the interpreter.
The examples are the same adopted for JavaScript. Therefore, you can compare languages, and notice similarities and differences between them.
Getting to Know Python Through Experimentation
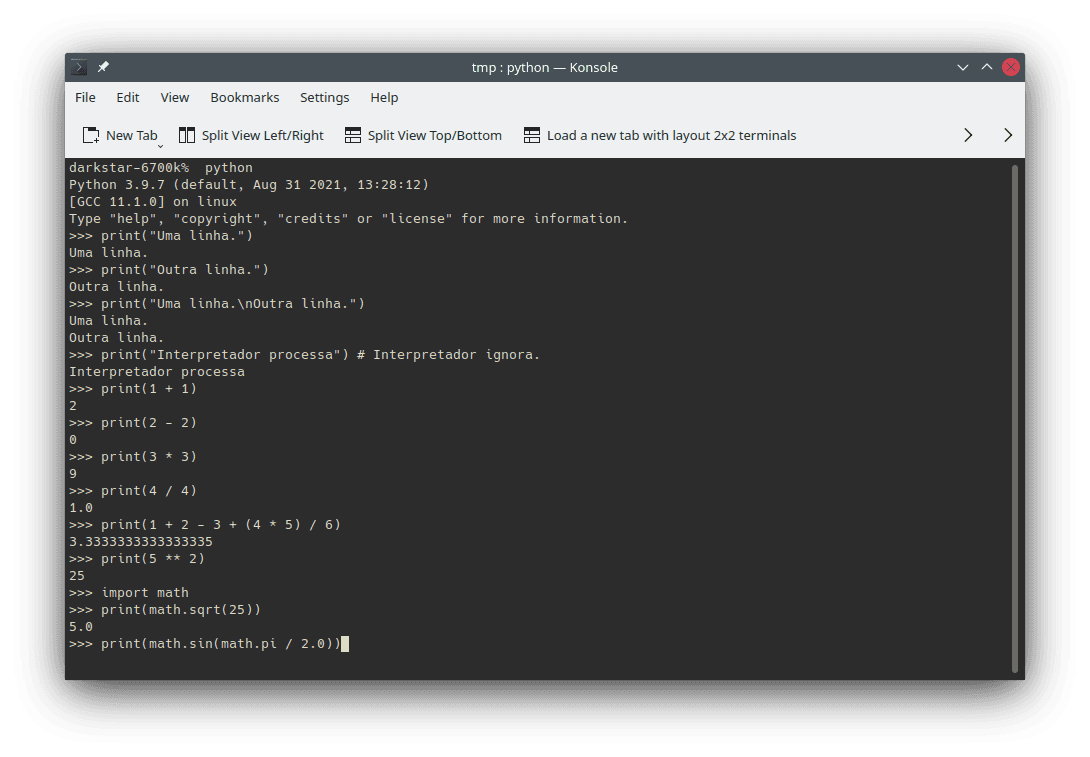
Text writing:
print("One line.") print("Another line.") print("One line.\nAnother line.")
Comments (text that is ignored by the interpreter; documentation):
print("Interpreter processes") # Interpreter ignores.
You can create comments spawning multiple lines in Python using a multi line string without assigning it to a variable.
""" <- Here the comment starts. It can spawn multiple lines. Here it ends -> """
""" It can also start and end in the same line. """
# Though, for single line comments, it is easier to use this comment style.
Mathematics operations:
Sum, subtraction, multiplication and division (documentation):
print(1 + 1) print(2 - 2) print(3 * 3) print(4 / 4) # What does happen if you try to divide by 0? Try it!
Math expressions:
print(1 + 2 - 3 + (4 * 5) / 6)
Power (documentation):
print(5 ** 2)
Square root (documentation: Math.sqrt()):
import math print(math.sqrt(25))
Trigonometric functions, such as sin (documentation: math.sin e math.pi):
import math # Unnecessary if you have imported it before in the same REPL session or source code file. print(math.sin(math.pi / 2.0)) # Sin.
Comparisons (result in
True
orFalse
):Equality (documentation):
print(1 == 2) # Equal: both equals are required! print(1 != 2) # Different.
print("Franco" == "Franco") print("Franco" != "Franco") print("Franco" != "Your name") # Python differs lower case characters from upper case ones (and vice-versa). print("F" == "f") print("F" != "f") print("Franco" == "franco") print("Franco" != "franco")
Other comparisons (documentation):
print(1 < 2) # Less than. print(1 > 2) # Greater than. print(1 <= 2) # Less or equal than. print(1 >= 2) # Greater or equal than.
Variables and assignment (documentation):
Variables are like boxes that store values put inside them. The assignment operator (a single equal sign (
=
) in Python) commits the storage.x = 123 print(x)
result = 123.456 + 987.654 print(result)
You should note that you can declare a variable with a chosen name only once. However, you can change its value as many times as you need.
name = "Franco" print(name) name = "Franco Garcia" print(name)
variables_can_vary = "Franco" print(variables_can_vary) variables_can_vary = "Seu Nome" print(variables_can_vary) variables_can_vary = "Outro Nome" print(variables_can_vary)
logical_value = True print(logical_value) logical_value = False print(logical_value) logical_value = (1 + 1 == 2)
Constants (documentation):
Python does not define constants, although there is a proposal to add them to the language.
from typing import Final PI: Final = 3.14159 print(PI)
from typing import Final E: Final = 2.71828 print(E) E = 0 # Error; the value of a constant cannot be changed once set.
Errors:
print(Ooops!) # Text should be between double quotes, single quotes, or grave accents. pri("Incorrect name for print") print(1 / 0) print(0 / 0)
Strings for words and text (documentation):
print("Ok, this is a valid string") print('Ok, this is another valid string') print("""Ok, this is also a valid string""")
print("If you want to 'mix' quotes, you have to use those different from the external ones.") print("Otherwise, you will need to escape them with a backslash, like this: \". The backslash is required.")
Logical operators (documentation):
print(True and True) # This is a logical "and". print(True or False) # This is a logical "or". print(not True) # This is a logical "not".
Conditions (documentation):
In Python, indentation (the spacing before the first symbol of a line of code) is important, because it defines the level of a source code block or scope. Thus, when there are spaces before the first character of a line, they must be written in the interpreter. You can use any number of spaces and tabs, provided that you are consistent. For instance, if the first line with spaces has four spaces, you will need to use four spaces in all lines belonging to the block.
browser = "Firefox" if (browser == "Firefox"): print("Mozilla Firefox.")
my_browser = "Your Browser" if (my_browser == "Firefox"): print("You use a browser by Mozilla.") else: print("You use another browser.")
i_use = "X" i_use = i_use.lower() if (i_use == "firefox"): print("You use a browser by Mozilla.") elif ((i_use == "chrome") or (i_use == "chromium")): print("You use a browser by Google.") elif (i_use == "edge"): print("You use a browser by Microsoft.") elif (i_use == "safari"): print("You use a browser by Apple.") elif (i_use == "internet explorer"): print("You should use a more modern browser...") else: print("You use another browser.")
Loops (documentation:
while
efor
):for i in range(5): print(i)
j = 0 while (j < 5): print(j) j += 1 # The same as j = j + 1
Python does not provide a
do... while
command. The following code is an equivalent version, although whether one should usebreak
is arguable. To avoid usingbreak
, it is possible to create a version withwhile
that duplicated the first line of code (or refactor the duplicated code into a function).k = 0 while (True): print(k) k += 1 if (k == 5): break
Functions (documentation):
def my_function(x, y): result = x + y return result # A function is a block of code that performs arbitrary processing as defined # by the programmer. # After the definition, you can execute the function whenever wanted, using # a function call. z = my_function(12, -34) # This is an example of a function call. print(z) print(my_function(1, 2)) # This is another example.
Data types (documentation):
integer_number = 1 another_integer_number = -1 real_number = 1.23 logic_value = True # or False; it only can be either True or False. string_for_text = "Text here. Line breaks use\nthat is, this will be at\n the third line."
Input (documentation: input() e int()):
To use this example in REPL, you must type (or copy and paste) one line at a time. This is required due to the use of
input()
, which will ask for the input of a value. You should type a value and pressenter
to send it to the interpreter. If you define a script, this is not necessary; the file can store the entire code for the program.# Once there is a request for a value, type one then press `enter`. your_name = input("What is your name? ") # int() converts a number in text format to a whole number. your_age = int(input("How old are you? ")) print(your_name) print(your_age) # The + operator for strings is not a sum, but an operation called # concatenation that combines the second string after the first. print("Hello, " + your_name + "!") # Prefixing a string with f allows converting variables between curly brackets # to strings. print(f"You are {your_age} years old.")
Congratulations! You Are Already Able to Write Any Program in Python
Don't you believe it? You can find the explanation in the introduction to JavaScript
In short, it is not enough to learn the syntax of a language to solve problems using it. It is much more important learning programming logic and developing computational thinking skills than learning the syntax of a programming language. In fact, when you are in doubt about the syntax, you just have to consult the documentation. You do not have to memorize it.
After you learn a first programming language, it is relatively simple to learn other languages (that adopt similar programming paradigms to the first one). To verify this statement, you can open a new window in your browser and place it side by side with this one. Then, you can compare the source code blocks written in Python (in this window) and in JavaScript (in the second window).
If you wish to perform comparisons with other programming languages, the following options are available:
Next Steps
Once the development environment is configured, you can continue your system development journey.
To avoid repetitions, I recommend reading the page about configuring the JavaScript environment. Even if you are not interested at the language, many discussions are relevant to any programming language. Besides, you will understand a way to create Internet pages and will be able to create content for your browser.
Until GDScript (for Godot Engine), the topics describe develoment environment configurations for a few programming languages. After GDScript, the focus becomes basic concepts for learning programming.