Development Environments: Java Programming Setup
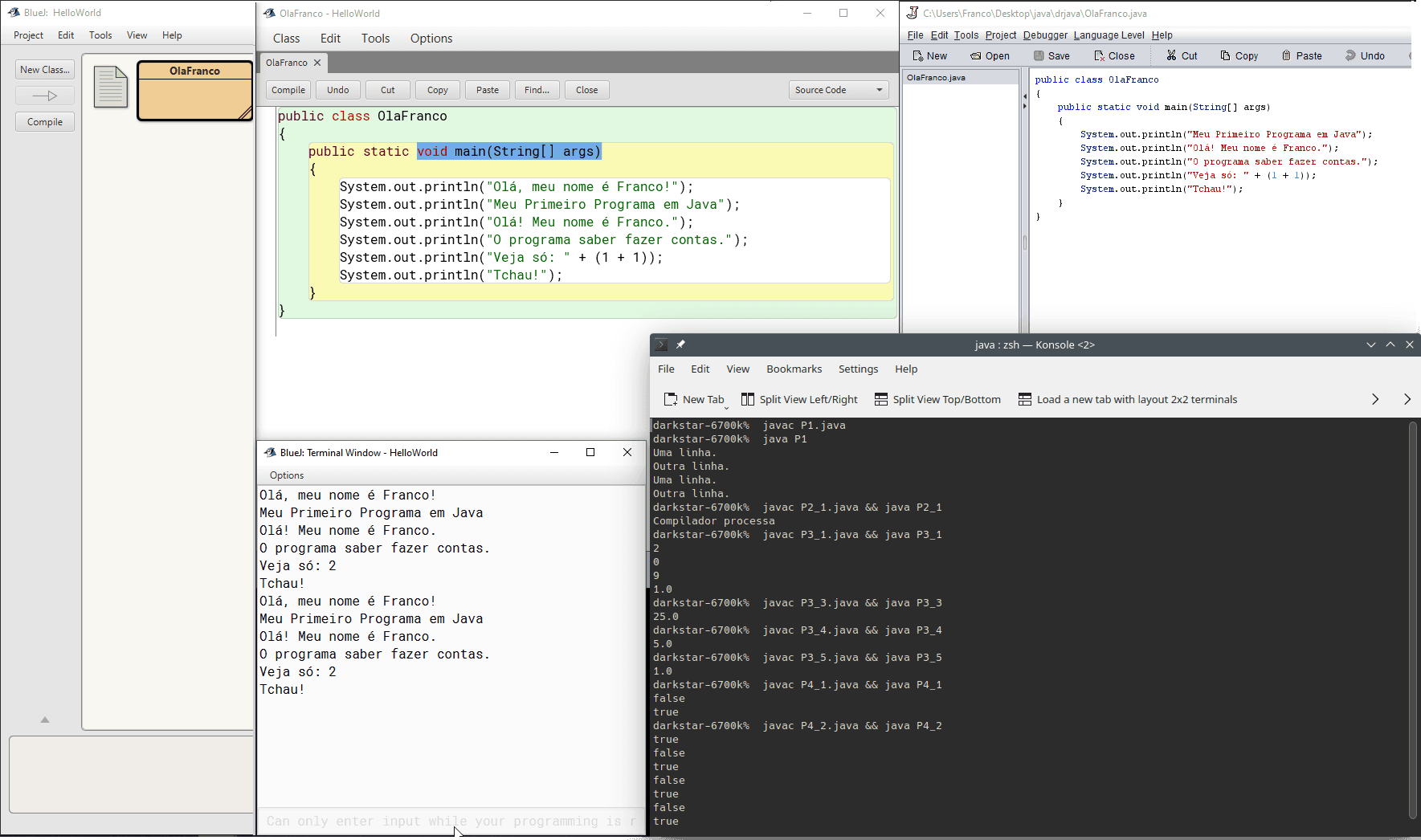
Image credits: Image created by the author using the program Gimp.
Requirements
The development environment configuration to start programming are similar to those described for the C programming language: a text editor and a compiler, or an Integrated Development Environment (IDE). For Java, you should install the Java Development Kit (JDK) to get the compiler. It is important paying attention to the acronym JDK, so you do not install the Java Runtime Environment (JRE) instead, which does not allow creating Java programs.
To avoid repeating the discussions from JavaScript and Python:
- If you already have a text editor, it suffices to install the JDK. However, it can be preferable to use an IDE when programming in Java. The IDEs for the language often provide excellent support for programming.
- If you would rather use an IDE, the simplest option is choosing either BlueJ or Greenfoot, which includes a text editor, JDK and debugger among other features;
- If you do now know how to install program, what is
PATH
or how to configure it, you can refer the topic regarding how to install programs. The topic can be particularly useful for Java because it is possible to install the JDK with Ninite. If you are not experienced installing programs, Ninite can simplify the process.
Part of the contents of this page will be identical to the Python's page. Therefore, if certain paragraphs seem familiar, it will not an impression, but déjà vu. As a matter of fact, this will also occur in new entries about development environments for other programming languages. After all, the setup procedures are similar.
Java as the First Programming Language
Python and Lua lead my list of recommended programming languages for beginners, as previously commented in introduction about development environments; the third option is JavaScript, due to the Internet.Although the previous languages can be simpler for beginners, Java can also be a good option to start learning programming. A strong reason is that many universities with Computing courses (such as Computer Science, Computer Engineering and Information Systems) use the language either in introductory classes or as part of the curriculum. Consequently, there are multiple IDEs and tools to support learning the language. The IDEs BlueJ, DrJava and Greenfoot are three examples.
If you do not want to use an IDE (or if the IDE requires manually installing the SDK), it is also easier to set up the JDK than a compiler for C and C++ (even on Windows).
Furthermore, Java is the default language to create applications for the Android operating systems. Although the complexity of programming for Android can be incompatible to beginners' skills, the transition is possible after acquiring some experience with Java. Naturally, it should be noted that the applications must be simple to accommodate the needs of the beginners. Nevertheless, the possibility of programming for mobile devices can serve as additional motivation to learn programming.
About Java
Like Python, Lua e JavaScript, Java is a high-level language. In other words, the language provides abstractions, features and resources that allow programmers to focus on solving problems instead of worrying about how the hardware works.
Unlike the previous languages, Java is, at its core, an object-oriented language (following the Object-Oriented Programming or OOP paradigm). Thus, Java variables are often objects. Types are normally classes. Discussions regarding OOP will have their own topics in the future. At this moment, an observable implication is that Java examples will be slightly different from those provided for other languages.
Java is a compiled and interpreted language. At a first moment, the source code is compiled as an intermediate representation called bytecode. At a second moment, the bytecode is interpreted by a Java Virtual Machine (JVM). Some JVM implementations may compile the bytecode when the program is being run, using a technique called Just-in-Time (JIT).
The intermediate representation can make Java programs portable for the platform that will run the code. As a result, one of Java's slogans is write once, run anywhere. Thus, like interpreted languages, it is possible to use a program without modifications if there exists a JVM implementation for the platform that will run the code.
In fact, portability is a highlight of Java. Java systems can be used in computer, in the Internet (Web, commonly for back-end), mobile devices and in embedded devices. Technologies such as servlets, Java Server Pages (JSP), applets (currently deprecated) and JavaFX allow using Java in browsers and Web servers.
Besides, Java is the main developing language for Android, which makes it one of the most relevant programming languages for mobile devices. Nowadays, Kotlin is a second option to develop for the platform. Nevertheless, as Android's Application Programming Interfaces (APIs) are written in Java, the use of the language can be required for some tasks or integration with the operating system.
Java can be a good option for beginners, due to its extensive and high quality standard library. Furthermore, as higher education (such as universities) often teach the language, there exists support tools to help learning the language (such as IDEs designed for beginners).
For documentation, the official source is Oracle's documentation.
Versions and Distributions
Before starting programming in Java, it is wise to know two acronyms: Java Runtime Environment (JRE) e Java Development Kit (JDK). This is important because you must install the JVM to program in Java. JRE allows using Java applications (for instance, by end-users), though it does not provide the development tools that programmers need to create programs using the language.
Furthermore, there exists three main distributions for the JDK:
- The first is the official JDK, maintained by Oracle;
- The second is the OpenJDK;
- The third is the AdoptOpenJDK, an alternative implementation of the OpenJDK. The project is being re-branding to a new name (Adoptium), maintained by the Eclipse Foundation (that also maintains the Eclipse IDE).
Any distribution is valid to start programming in Java. However, some distributions can be more (or less) popular for some operating systems. For instance, OpenJDK is usually available on package managers of on Linux distributions.
There are other JDK distributions, such as Red Hat's, Microsoft's and Amazon's (Amazon Corretto).
In general, I would recommend beginners to choose either OpenJDK or AdoptOpenJDK. For professional use, the ideal scenario is analyzing licenses, implementation, support, and stability of each project before choosing one. For instance, depending on the project requirements, it can be preferable to use the most up-to-date distribution, or the one with the best long-term support (LTS).
Compiler and Text Editor
You should use root
or administrator
accounts only to install the compiler.
After the installation, use a regular user account, with lower privileges, to run the compiler and program using it.
You should also run your created programs with a regular user account.
Once again, it is important taking care to install the JDK, not the JRE.
Linux
The following examples describe how to install the JDK in a few Linux distributions. To install other JDK versions, check the provided documentation.
- Arch Linux:
pacman -S jdk-openjdk
- Debian, Ubuntu or distributions based on the former ones:
apt-get install default-jdk
- Fedora:
dnf install java-latest-openjdk-devel.x86_64
- Gentoo:
emerge --ask --oneshot virtual/jdk
macOS
On macOS environments, you can use brew
to install the OpenJDK.
Windows
One option to install Java for Windows is using Ninite. As Ninite provides the AdoptOpenJDK, the following steps for Windows also adopt it.
As the project migrated to Adoptium, you can access the page, choose a version and download the installer. When this topic was written, the available versions where Temurin 8 (LTS), Temurin 11 (LTS), Temurin 17 (LTS), corresponding, respectively, to the versions 8, 11 and 17 of the JDK. The default choice was version 17, which could be chosen by clicking on Latest release.
Any version is valid for learning purposes.
Though if you want to use the DrJava
IDE, you must choose the version 8 (Temurin 8 (LTS)).
Otherwise, you can choose the most recent version, as Temurin 17 (LTS).
When running the JDK, it is convenient enabling the options Add to Path
(enabled by default), Associate to .jar
(enabled by default), and Set JAVA_HOME variable
(disabled by default).
From the three, the addition to the PATH
is the most important option.
The other two are convenient.
The file association with .jar
allows running Java's .jar
files with a double click.
JAVA_HOME
defines the JRE directory, to run programs written in Java.
If you already have a JRE installed, you can ignore it.
To enable the tree options, click the disk icon next to each entry and choose Will be installed on local hard drive.
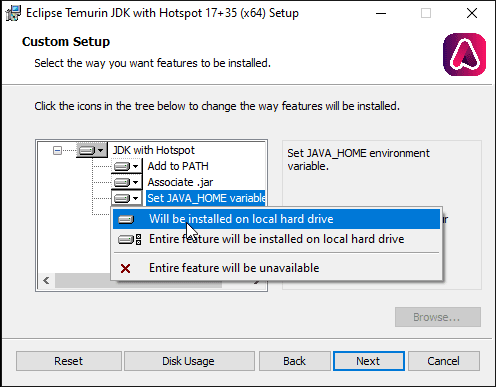
After the set-up finishes, the commands java
and javac
should be available in command interpreters such as cmd
.
If you speak a non-English language and wish to use accents in cmd
, Java source code files (.java
) should be encoded in one of the Windows codifications (for instance, windows-1252
for Latin derived languages, such as Brazilian Portuguese).
In programming text editors, the previous codification should be available as Western European (Oeste Europeu) / Windows-1252
.
Other text codifications, such as UTF-8, will probably provide wrong characters in cmd
.
Alternatives to install the JDK on Windows include:
- Ninite, Chocolatey or Scoop, as previously discussed, including `PATH` configuration;
- An IDE with an integrated JDK (for instance, BlueJ or Greenfoot).
Environment Test
You can start to program in Java after installing the JDK (or an IDE with the JDK). The process is similar to the one described for the C programming language in Compilers and Program, though it is (usually) simpler.
This section presents the same examples used in JavaScript, Python and Lua for purposes of testing and comparing the languages.
To start, you can create a file with the following contents (System.out.println()
is a method from the standard library to write in console; documentation):
public class OlaFranco
{
public static void main(String[] args)
{
System.out.println("Olá, meu nome é Franco!");
}
}
"Olá, meu nome é Franco!" means "Hello, my name is Franco!" in Portuguese.
In Java, the filename must match the class name.
Thus, in the previous case, the filename must be OlaFranco.java
.
Next, you can compile the file:
javac OlaFranco.java
The result will be a file called OlaFranco.class
, storing bytecode.
To run the program, you will an interpreter:
java OlaFrancoOlá! Meu nome é Franco.
It should be noted that the command uses only the name of the class (OlaFranco
) instead of the name of the generated file.
An attempt to run the file OlaFranco.class
would result into an error.
Likewise, you should not run java OlaFranco.java
; you should compile the file first.
If the previous two points are considered, the compilation and interpretation should succeed.
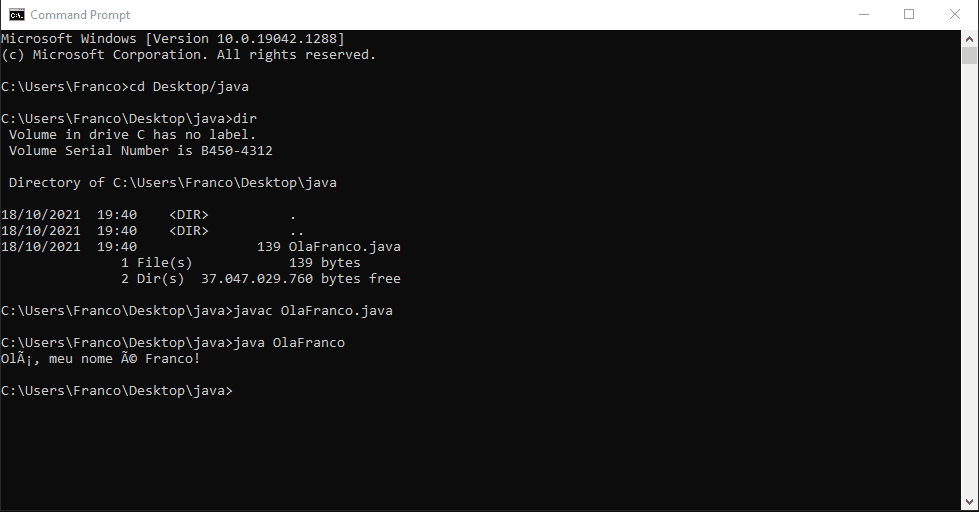
Once again, the encoding is relevant to use accents on cmd
.
If the file is encoded using UTF-8, accents will not work.
If you want to use accents, configure the text editor to use something like windows-1252
for Latim derived languages (such as Portuguese).
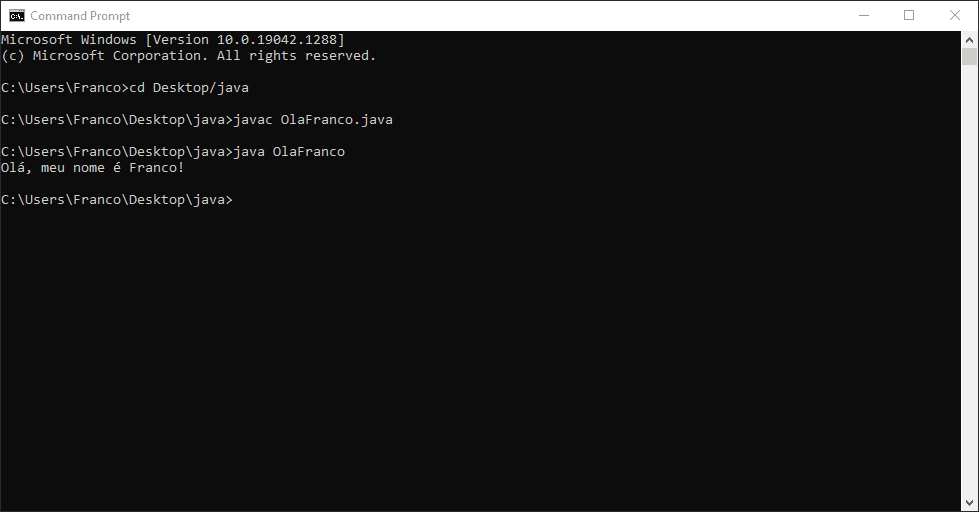
Unlike C and C++, the process is the same for all operating systems. Accents should also work without further configuration (at least for systems with support for Brazilian Portuguese).
Then, to keep the tradition, you can create your second Java program.
To further keep the tradition for the first script filename, the file can be called FirstProgram.java
.
Thus, the class must be called FirstProgram
.
If you wish, you can rename both to SecondProgram
.
public class PrimeiroPrograma
{
public static void main(String[] args)
{
System.out.println("My First Java Program");
System.out.println("Hello! My name is Franco.");
System.out.println("The program can do Math.");
System.out.println("Check it out: " + (1 + 1));
System.out.println("Bye!");
}
}
javac FirstProgram.javajava FirstProgramMy First Java Program
Hello! My name is Franco.
The program can do Math.
Check it out: 2
Bye!
Congratulations! If you have gotten to this point, now you understand how an IDE compiles and run programs behind the scenes. The next section shows how IDEs make the process easier, especially for beginners.
IDEs
The same considerations about the use of IDEs in Python apply to Java.
An IDE makes it easier to compile and run programs under development. If you are not familiar with the command line, an IDE will help you to focus on activities to learn programming instead of how to use a shell.
Thus, the option to run code with a single click is very convenient.
In particular, search for the option to run a project with a single click (normally on a triangle icon (🢒
) commonly used to play content eletroelectronics) or with a key press (usually F5
).
Furthermore, remember shortcuts for text editors, such as Ctrl n
(or File / New
) to create new files and Ctrl o
(or File / Open
) to open existing files.
Like Thonny for Python, Python has IDEs that were designed for beginners. BlueJ, Greenfoot and DrJava are three examples.
BlueJ
A first option for programming beginners is BlueJ, maintained by the King's College London (England). The set-up options for Windows include a JDK.
BlueJ
includes support to Unified Modeling Language (UML), allowing one to visualize the initial modeling of the system under development, as well as the program's structure and relations among classes of the Java project.
Program structuring is important for software architectures.
Other BlueJ
resources for beginners include:
- Visual separation of the code in blocks;
- Visual inspection of objects when debugging;
- A panel to evaluate and test code.
To create a project using BlueJ
:
- Click on Project, then on New Project;
- Choose a name and directory for the project;
- Confirm;
- Click on New Class;
- Choose a name for the class (for instance,
OlaFranco
). The checked options should beJava
andClass
. - Double-click on the icon generated for the class (
OlaFranco
). Alternatively, you can right-click it to open the context menu, then chooseOpen Editor
. - Write the source code;
- Click on
Compile
; - To run the project, right-click the icon with the name of the class (
OlaFranco
) and choosevoid main(String[] args)
.
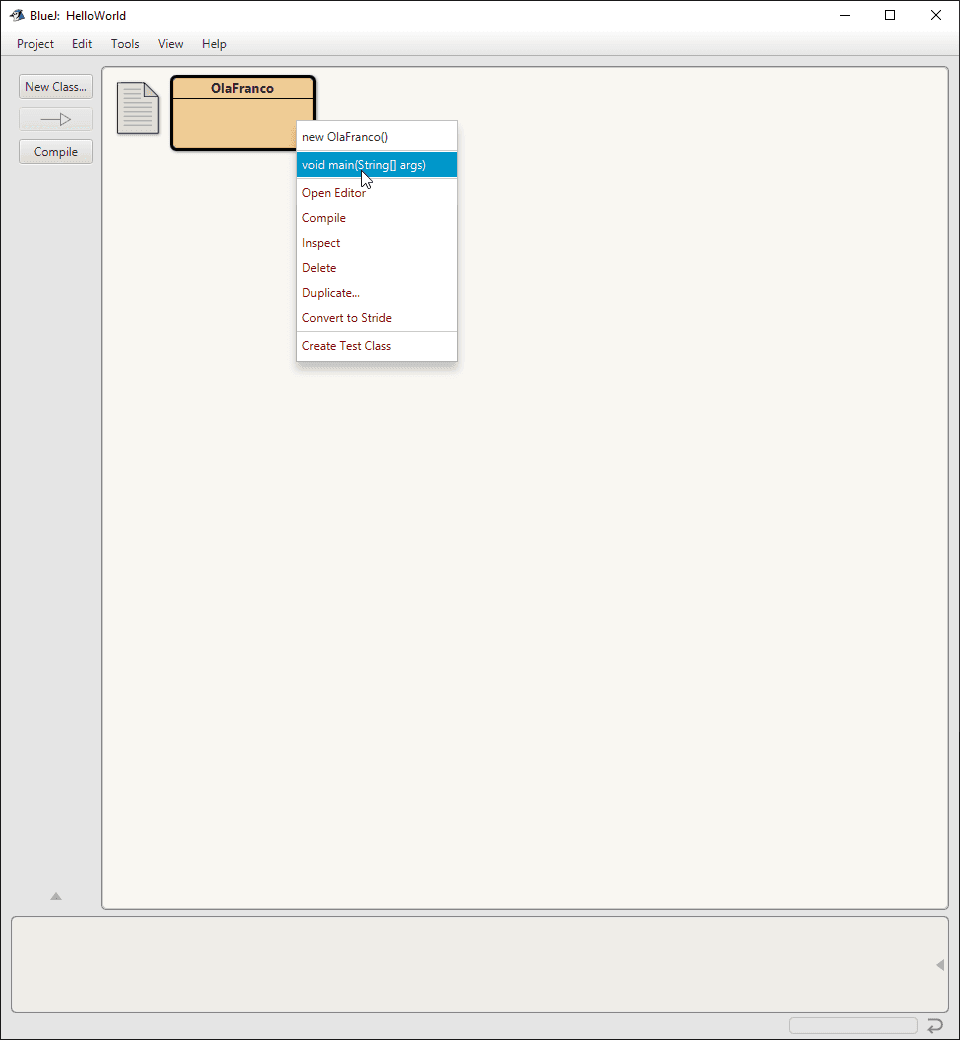
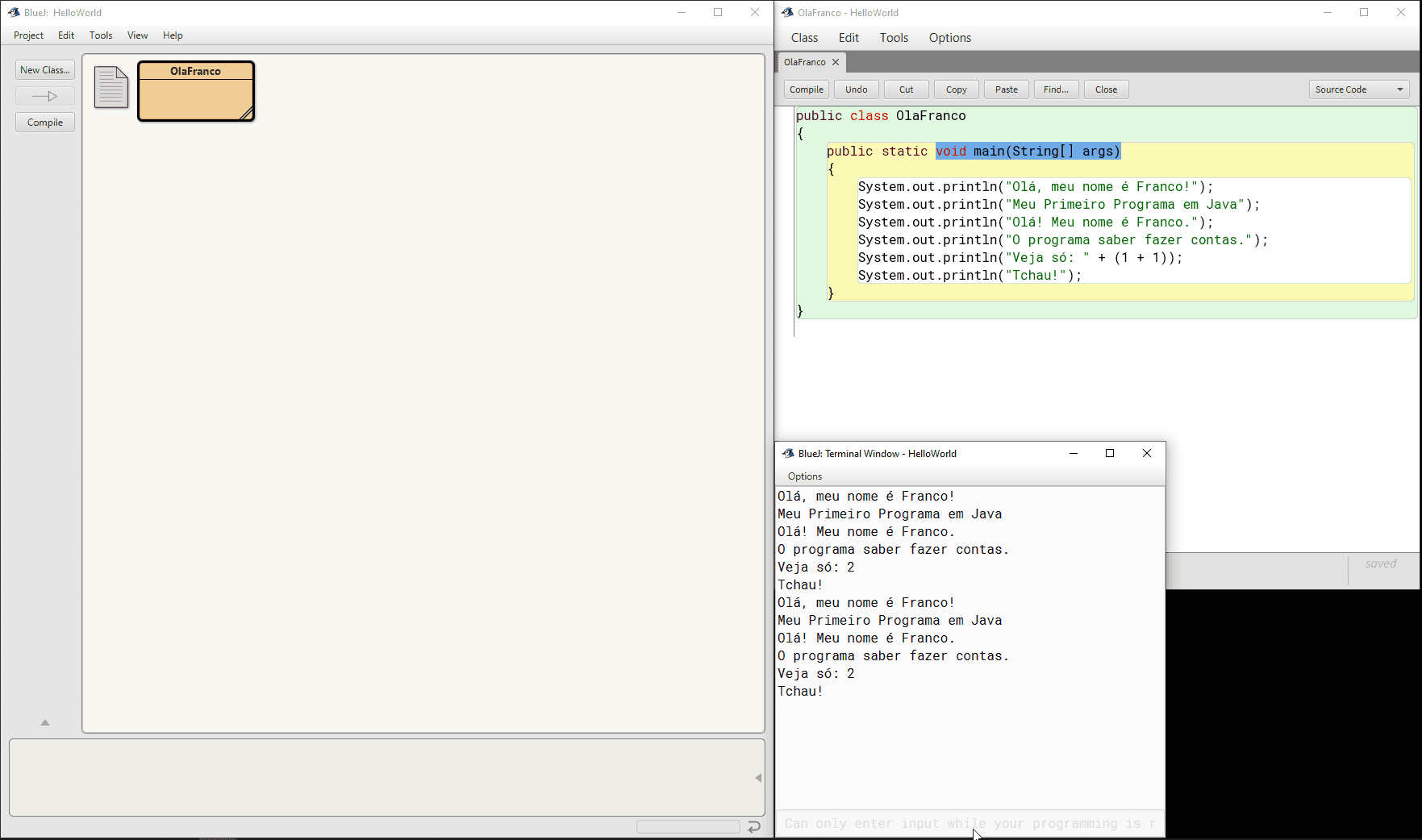
If you want to use a translation for IDE:
- Click on Tools, then on Preferences;
- Click on Interface;
- Choose the desired language (for instance, Portuguese);
- Click on OK;
- Restart the IDE (close it and open it again).
In Interface, it is also possible to enable the accessibility mode, which provides support for screen readers (for instance, for blind people or with other visual disability).
Greenfoot
A section option (which is related to Blue
IDE) is Greenfoot, maintained by the University of Kent (England).
Like BlueJ
, Greenfoot
for Windows include a JDK.
Greenfoot
shares some resources with BlueJ
, though it provides additional features designed mainly for younger people.
The IDE also provides an environment to create simulations using images, combing the traditional textual programming with elements of visual programming, swapping the use of UML by a board metaphor.
To create a project using Greenfoot
:
- Click on Scenario, then on New Java Scenario...;
- Choose a name and directory for the project;
- Confirm;
- Click on Edit, then on New Class...;
- Choose a name for the class (for instance,
OlaFranco
). The checked option should beJava
. - Double-click on the icon generated for the class (
OlaFranco
). Alternatively, you can right-click it to open the context menu, then chooseOpen Editor
. - Write the source code;
- Click on
Compile
; - To run the project, right-click the icon with the name of the class (
OlaFranco
) and choosevoid main(String[] args)
.
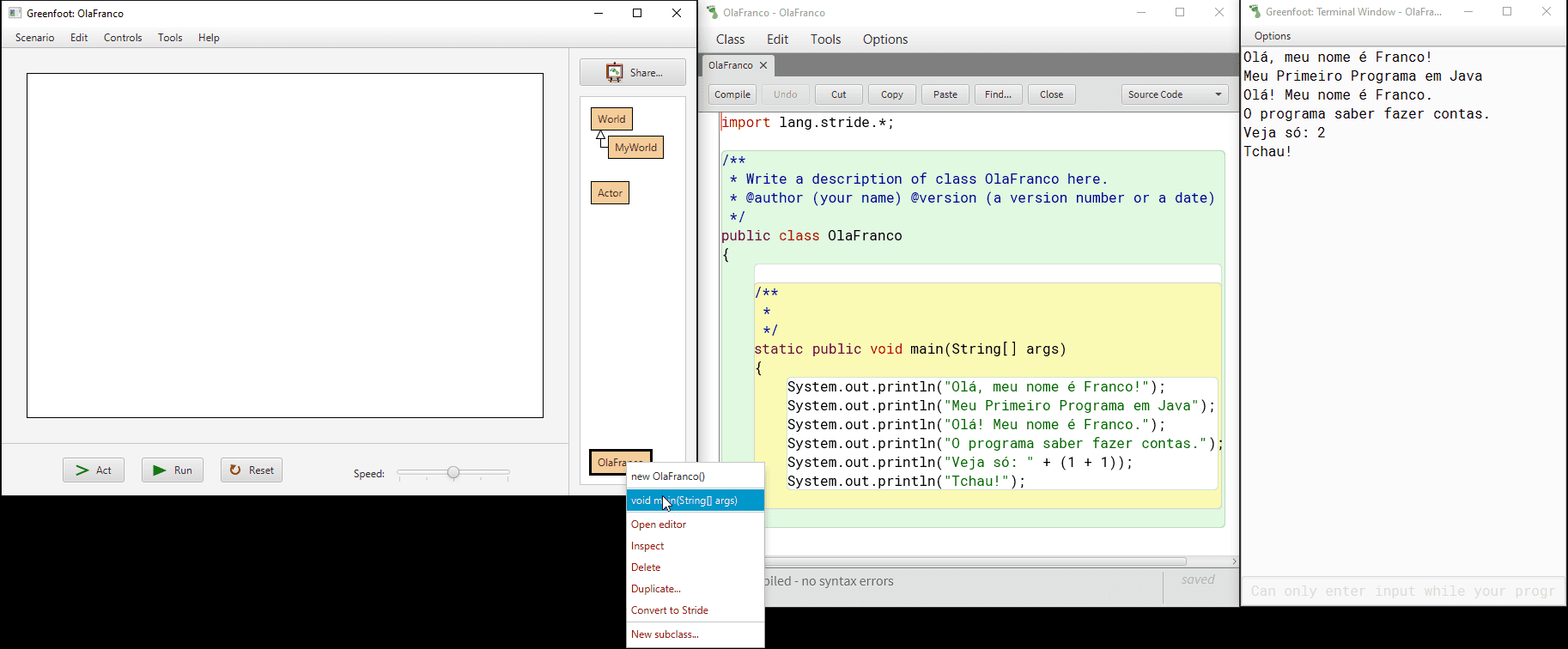
Caso queira utilizar o IDE em Português:
If you want to use a translation for IDE:
- Click on Tools, then on Preferences;
- Click on Interface;
- Choose the desired language (for instance, Portuguese);
- Click on OK;
- Restart the IDE (close it and open it again).
In Interface, it is also possible to enable the accessibility mode, which provides support for screen readers (for instance, for blind people or with other visual disability).
DrJava
The third option for beginners is DrJava, maintained by the Rice University (United States of America).
DrJava
resembles a traditional IDE, though with a simpler graphical user interface.
Essential features to edit, compile, and debug projects are available.
As the IDE does not include a JDK in the installer, you should configure the JDK before using the IDE.
Thus, if the IDE does not work (it is a .jar
file, a package with a program for the JRE) or if you cannot compile your projects, you should check if you have an installed JDK.
If you need the JDK, you should install the JDK 8.
If you use a newer version, the compilation and execution will fail with a message Current document is out of sync with the Interactions Pane and should be recompiled!.
If there exists a file association for .jar
files to use Java, you can start the IDE by double-clicking on it.
Otherwise, you will need to run the following command:
java -jar drjava-beta-20190813-220051.jar
The numbers or the filename itself can vary according to the version of the IDE.
In the first run on Windows system, you may receive an alert about an attempt to use the network.
If you want to use DrJava
's debugger, you need to allow the IDE to use the network (more information on the frequently asked questions and the answer in this link).
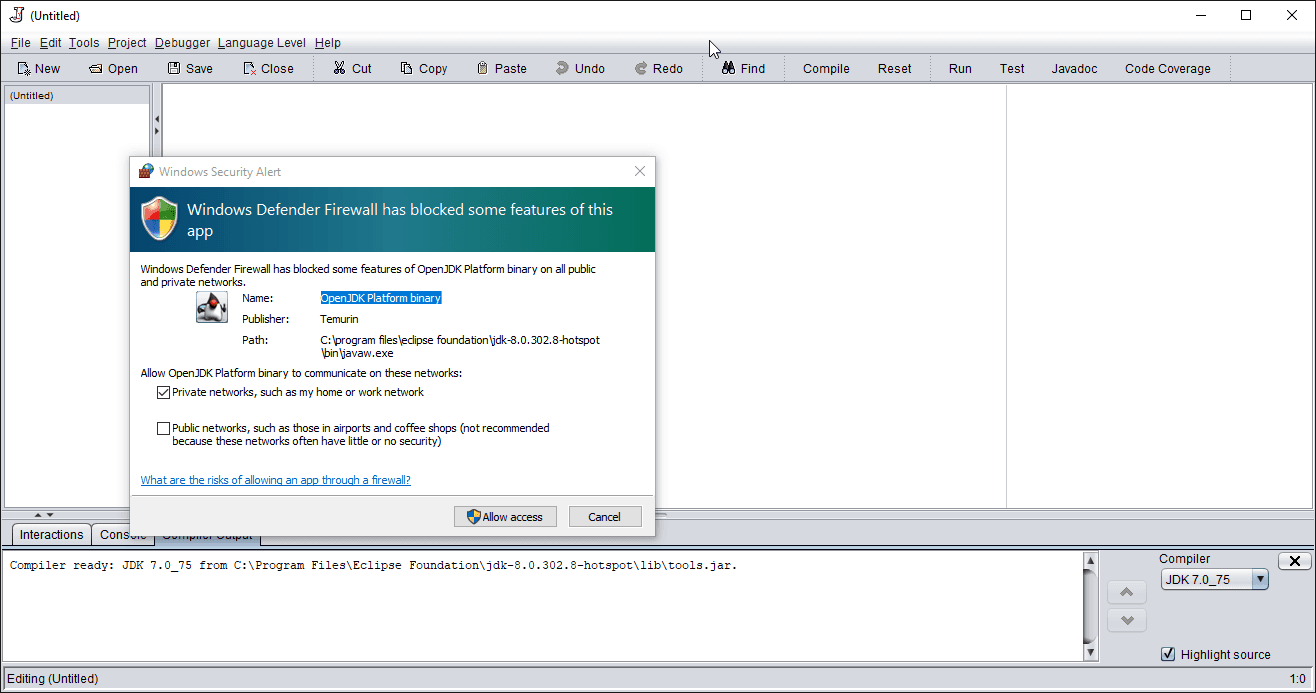
To create a project with DrJava
:
- Click on New (or File, then New);
- Type the source code in the new file;
- Save the file (Save) with a name and the
.java
extension (for instance,OlaFranco.java
); - Click on Compile;
- Click on Run.
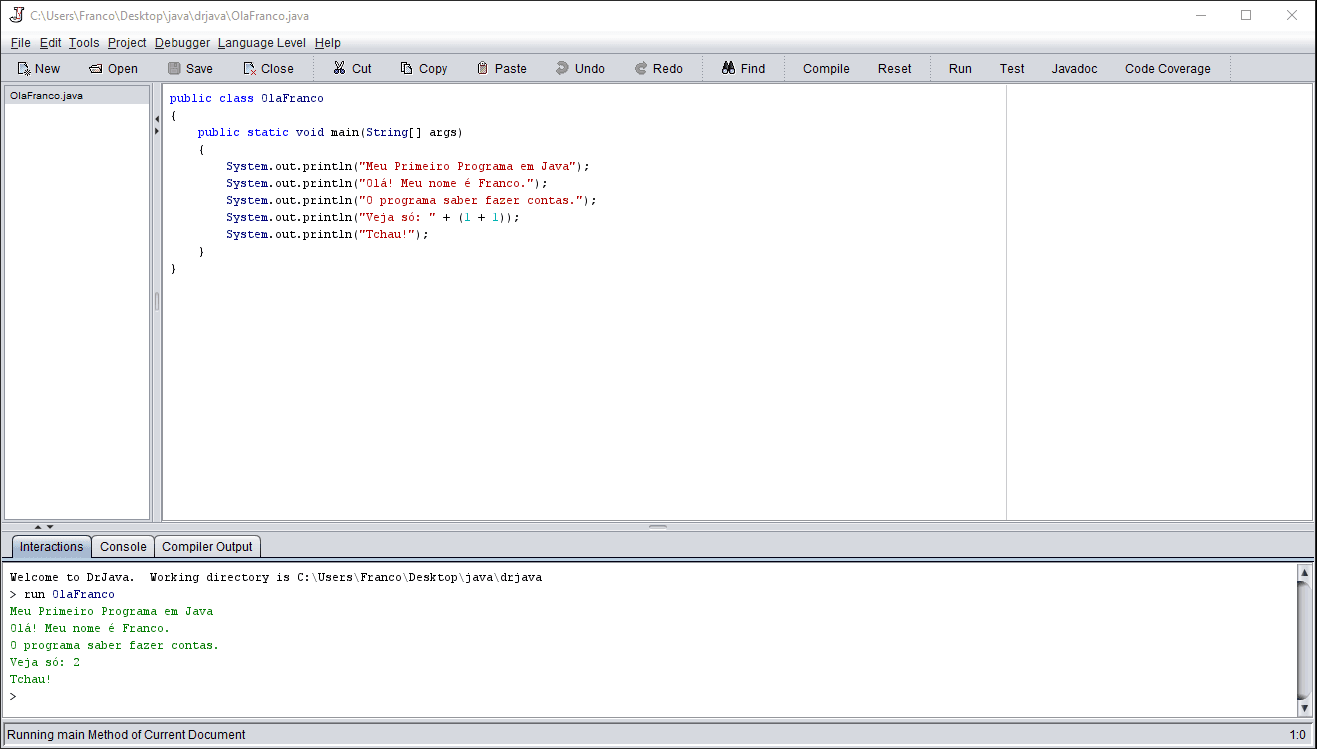
NetBeans
A traditional IDE for Java is called NetBeans.
NetBeans
includes the JDK, as well as further resources for advanced Java programming.
The IDE is more complete than the options for beginners, although it is still simpler to use than IDEs such as Eclipse
e IntelliJ IDEA
.
NetBeans
include features such as:
- Refactoring;
- Code generation for inherited methods;
- Application server to use Java with Web systems (for instance, with JSP).
Eclipse
The Eclipse IDE is a more complex alternative to Netbeans
, though with additional resources.
Eclipse
is a traditional option for academic and professional use.
For many years, Eclipse
has been one of the most complete options for Java development.
In particular, there are projects that use the IDE as an infrastructure for new development resources, test and aid on the comprehension of projects using Java.
IntelliJ IDEA
The IntelliJ IDEA has become a popular option for Java development during the last years.
Unlike the previous options, IntelliJ IDEA
is a proprietary IDE.
The Community Edition is free, though it is not as complete as Eclipse
or NetBeans
.
For learning programming, the free version is enough for most scenarios.
However, if you wish to program Java for the Web, Eclipse
and NetBeans
can be more convenient.
If you would rather use IntelliJ IDEA
, an option is using it along another IDE to use features that are missing in the Community Edition.
In particular, I would not recommend beginners to purchase a professional license to use the IDE.
However, students may apply for a educational license.
Other Options
There are many other Java IDEs for Java, among which we can cite:
- Xcode;
- jGRASP (if you want to use UML diagrams);
- Android Studio (if you want to start programming for Android).
First Steps to Begin Programming in Java
The following source code snippets illustrate some resources of the Java programming language. At this time, it is not necessary to fully understand them; the purpose is showing resources and the syntax of the language.
There are two ways of using the code. You can:
- Write and run the code in the IDE of your choice;
- Write the code using a text editor, generate a program using the file with code in a compiler, and run the generated program in your computer.
This simplest and fastest way is to use an IDE.
The examples are the same adopted for JavaScript. Therefore, you can compare languages, and notice similarities and differences between them.
Getting to Know Java Through Experimentation
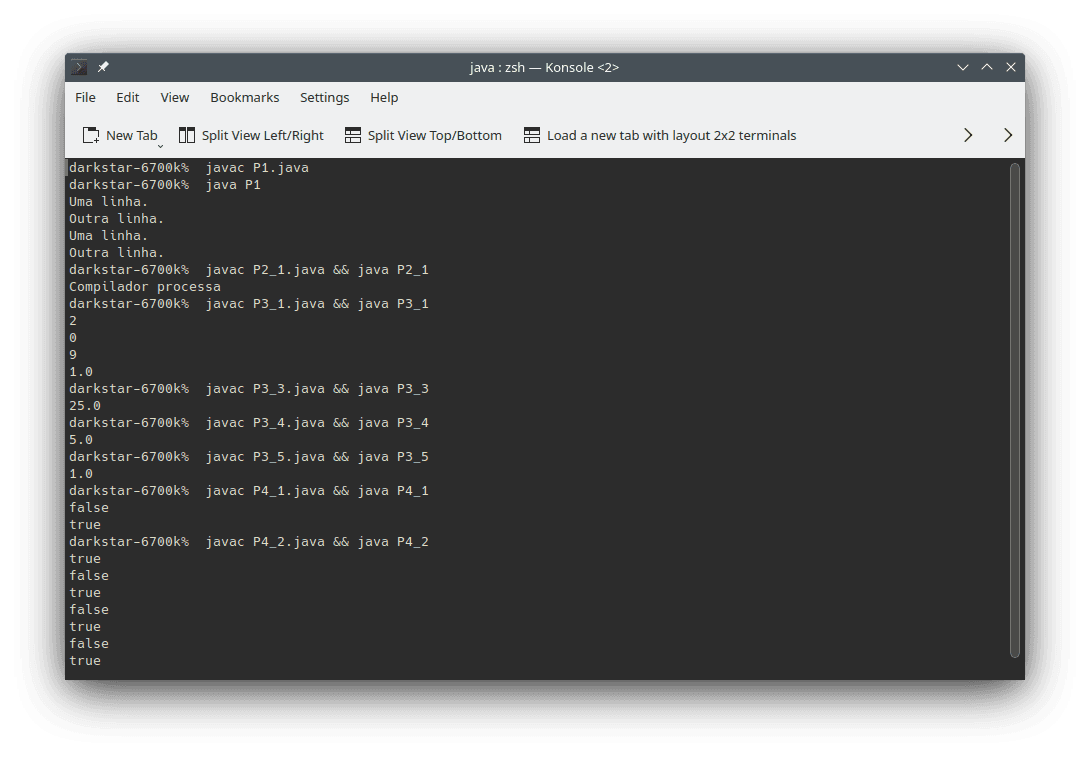
Text writing:
// javac P1.java && java P1 public class P1 { public static void main(String[] args) { System.out.println("One line."); System.out.println("Another line."); System.out.println("One line.\nAnother line."); } }
To compile and run without an IDE, create a file named
1.java
with the previous contents and type:javac P1.java java P1
You can choose other name for the source code file (
.java
) and for the executable to be compiled. If you change the file name, you must also rename the class with the new name. The name cannot contain spaces, accented characters or special symbols, neither start with a number.In the next examples, the compilation line will be commented in the first line of the source code.
If you do not want to create a file for each example, you can erase the contents and write the code from the next example. If you do want to save a copy of each example, (at this moment) it is necessary to create individual files.
Comments (text that is ignored by the interpreter; documentation):
// javac P2_1.java && java P2_1 public class P2_1 { public static void main(String[] args) { System.out.println("Compiler processes"); // Compilador ignores. } }
// javac P2_2.java && java P2_2 public class P2_2 { public static void main(String[] args) { /* <- Here the comment starts. It can spawn multiple lines. Here it ends -> */ } }
// javac P2_3.java && java P2_3 public class P2_3 { public static void main(String[] args) { /* It can also start and end in the same line. */ } }
// javac P2_4.java && java P2_4 public class P2_4 { public static void main(String[] args) { // Though, for single line comments, it is easier to use this comment style. } }
Mathematics operations:
Sum, subtraction, multiplication and division (documentation):
In Java, the division of two integer numbers result in an integer number (the remainder is truncated). To perform a real number division, at least one of the numbers must be real. For instance, compare the values of the divisions
6 / 4
and6 / 4.0
.
// javac P3_1.java && java P3_1 public class P3_1 { public static void main(String[] args) { System.out.println(1 + 1); System.out.println(2 - 2); System.out.println(3 * 3); System.out.println(4 / 4.0); // What does happen if you try to divide by 0? Try it! } }
- Math expressions:
// javac P3_2.java && java P3_2 public class P3_2 { public static void main(String[] args) { System.out.println(1 + 2 - 3 + (4 * 5) / 6.0); } }
- Power (documentation: Math.pow()):
// javac P3_3.java && java P3_3 import java.lang.Math; public class P3_3 { public static void main(String[] args) { System.out.println(Math.pow(5, 2)); } }
- Square root (documentation: Math.sqrt()):
// javac P3_4.java && java P3_4 import java.lang.Math; public class P3_4 { public static void main(String[] args) { System.out.println(Math.sqrt(25)); } }
- Trigonometric functions, such as sin (documentation: Math.sin() and Math.PI):
// javac P3_5.java && java P3_5 import java.lang.Math; public class P3_5 { public static void main(String[] args) { System.out.println(Math.sin(Math.PI / 2.0)); // Seno. } }
Comparisons:
- Equality (documentation; String):
// javac P4_1.java && java P4_1 public class P4_1 { public static void main(String[] args) { System.out.println((1 == 2)); // Equal: both equals are required! System.out.println((1 != 2)); // Different. } }
// javac P4_2.java && java P4_2 public class P4_2 { public static void main(String[] args) { System.out.println("Franco".equals("Franco")); System.out.println(!("Franco".equals("Franco"))); System.out.println(!("Franco".equals("Seu Nome"))); // Java differs lower case characters from upper case ones (and vice-versa). System.out.println('F' == 'f'); // Single characters in C++ are integer numbers. System.out.println('F' != 'f'); System.out.println("Franco".equals("franco")); System.out.println(!("Franco".equals("franco"))); } }
- Other comparisons (documentation):
// javac P4_3.java && java P4_3 public class P4_3 { public static void main(String[] args) { System.out.println((1 < 2)); // Less than. System.out.println((1 > 2)); // Greater than. System.out.println((1 <= 2)); // Less or equal than. System.out.println((1 >= 2)); // Greater or equal than. } }
Variables and assignment (documentation):
Variables are like boxes that store values put inside them. The assignment operator (a single equal sign (
=
) in Java) commits the storage.// javac P5_1.java && java P5_1 public class P5_1 { public static void main(String[] args) { int x = 123; System.out.println(x); } }
// javac P5_2.java && java P5_2 public class P5_2 { public static void main(String[] args) { float result = 123.456f + 987.654f; System.out.println(result); } }
You should note that you can declare a variable with a chosen name only once. However, you can change its value as many times as you need.
// javac P5_3.java && java P5_3 public class P5_3 { public static void main(String[] args) { String name = "Franco"; System.out.println(name); name = "Franco Garcia"; System.out.println(name); } }
// javac P5_4.java && java P5_4 public class P5_4 { public static void main(String[] args) { String variables_can_vary = "Franco"; System.out.println(variables_can_vary); variables_can_vary = "Seu Nome"; System.out.println(variables_can_vary); variables_can_vary = "Outro Nome"; System.out.println(variables_can_vary); } }
// javac P5_5.java && java P5_5 public class P5_5 { public static void main(String[] args) { boolean logical_value = true; System.out.println(logical_value); logical_value = false; System.out.println(logical_value); logical_value = (1 + 1 == 2); } }
Constants (documentation):
final
does not exactly define constants in Java, for the value of the variable can change in some circumstances (for instance, for objects). The use offinal
allows initializing a variable only once. However, the use of methods can change the stored value (the language only does not allow to change the instance stored in a variable).// javac P6_1.java && java P6_1 public class P6_1 { final static float PI = 3.14159f; public static void main(String[] args) { System.out.println(PI); } }
// javac P6_2.java && java P6_2 public class P6_2 { final static float E = 2.71828f; public static void main(String[] args) { System.out.println(E); E = 0; // Error; the value of a constant cannot be changed once set. } }
Errors:
// javac P7.java && java P7 public class P7 { public static void main(String[] args) { System.out.println(Ooops!); // Text should be between double quotes. System.out.prin("Incorrect name for System.out.println"); System.out.println(1 / 0); System.out.println(0 / 0); } }
Strings for words and text (documentation):
// javac P8_1.java && java P8_1 public class P8_1 { public static void main(String[] args) { System.out.println("Ok, this is a valid string"); System.out.println("Ok, this is another valid string"); System.out.println("Ok, this is also a valid string"); } }
// javac P8_2.java && java P8_2 public class P8_2 { public static void main(String[] args) { System.out.println("If you want to 'mix' `quotes`, you have to use those different from the external ones."); System.out.println("Otherwise, you will need to escape them with a backslash, like this: \". The backslash is required."); } }
Logical operations (documentation):
// javac P9.java && java P9 public class P9 { public static void main(String[] args) { System.out.println(true && true); // This is a logical "and". System.out.println(true || true); // This is a logical "or". System.out.println(!false); // This is a logical "not". } }
Conditions (documentation):
// javac P10_1.java && java P10_1 public class P10_1 { public static void main(String[] args) { String browser = "Firefox"; if (browser.equals("Firefox")) { System.out.println("Mozilla Firefox."); } } }
// javac P10_2.java && java P10_2 public class P10_2 { public static void main(String[] args) { String my_browser = "Your Browser"; if (my_browser.equals("Firefox")) { System.out.println("You use a browser by Mozilla."); } else { System.out.println("You use another browser."); } } }
// javac P10_3.java && java P10_3 public class P10_3 { public static void main(String[] args) { String i_use = "X"; i_use = i_use.toLowerCase(); if (i_use.equals("firefox")) { System.out.println("You use a browser by Mozilla."); } else if ((i_use.equals("chrome")) || (i_use.equals("chromium"))) { System.out.println("You use a browser by Google."); } else if (i_use.equals("edge")) { System.out.println("You use a browser by Microsoft."); } else if (i_use.equals("safari")) { System.out.println("You use a browser by Apple."); } else if (i_use.equals("internet explorer")) { System.out.println("You should use a more modern browser..."); } else { System.out.println("You use another browser."); } } }
Loops (documentation: for, while, and do...while):
// javac P11_1.java && java P11_1 public class P11_1 { public static void main(String[] args) { int i; for (i = 0; i < 5; i = i + 1) { System.out.println(i); } } }
// javac P11_2.java && java P11_2 public class P11_2 { public static void main(String[] args) { int j = 0; while (j < 5) { System.out.println(j); ++j; // The same as j = j + 1 and similar to j++ (there are slightly differences) } } }
// javac P11_3.java && java P11_3 public class P11_3 { public static void main(String[] args) { int k = 0; do { System.out.println(k); k++; } while (k < 5); } }
Functions (documentation):
To be more precise, functions in Java are methods, because they belong to a class. To avoid OOP discussions, the following program defined a static method, that can be related to a function in other languages.
// javac P12.java && java P12 public class P12 { public static int my_function(int x, int y) { int result = x + y; return result; } public static void main(String[] args) { // A function is a block of code that performs arbitrary processing as defined // by the programmer. // After the definition, you can execute the function whenever wanted, using // a function call. int z = my_function(12, -34); // This is an example of a function call. System.out.println(z); System.out.println(my_function(1, 2)); // This is another example. } }
Data types (documentation):
// javac P13.java && java P13 public class P13 { public static void main(String[] args) { int integer_number = 1; int another_integer_number = -1; float real_number = 1.23f; boolean logic_value = true; // or false; it only can be either true or false. String string_for_text = "Text here. Line breaks use\nthat is, this will be at\n the third line."; } }
Input (documentation: BufferedReader.readLine(), InputStreamReader and Integer.parseInt()):
// javac P14.java && java P14 import java.io.*; import java.util.Scanner; public class P14 { public static void main(String[] args) throws IOException { BufferedReader line_reader = new BufferedReader(new InputStreamReader(System.in)); // Once there is a request for a value, type one then press `enter`. System.out.print("What is your name? "); String your_name; your_name = line_reader.readLine(); System.out.print("How old are you? "); int your_age; your_age = Integer.parseInt(line_reader.readLine()); System.out.println(your_name); System.out.println(your_age); System.out.println("Hello, " + your_name + "!"); System.out.println("You are " + your_age + " years old."); } }
Congratulations! You Are Already Able to Write Any Program in Java
Don't you believe it? You can find the explanation in the introduction to JavaScript
In short, it is not enough to learn the syntax of a language to solve problems using it. It is much more important learning programming logic and developing computational thinking skills than learning the syntax of a programming language. In fact, when you are in doubt about the syntax, you just have to consult the documentation. You do not have to memorize it.
After you learn a first programming language, it is relatively simple to learn other languages (that adopt similar programming paradigms to the first one). To verify this statement, you can open a new window in your browser and place it side by side with this one. Then, you can compare the source code blocks written in Java (in this window) and in Python (in the second window).
If you wish to perform comparisons with other programming languages, the following options are available:
Next Steps
Once the development environment is configured, you can continue your system development journey.
To avoid repetitions, I recommend reading the page about configuring the JavaScript environment. Even if you are not interested at the language, many discussions are relevant to any programming language. Besides, you will understand a way to create Internet pages and will be able to create content for your browser.
Until GDScript (for Godot Engine), the topics describe develoment environment configurations for a few programming languages. After GDScript, the focus becomes basic concepts for learning programming.