Learn Programming: Terminal (Console) Input
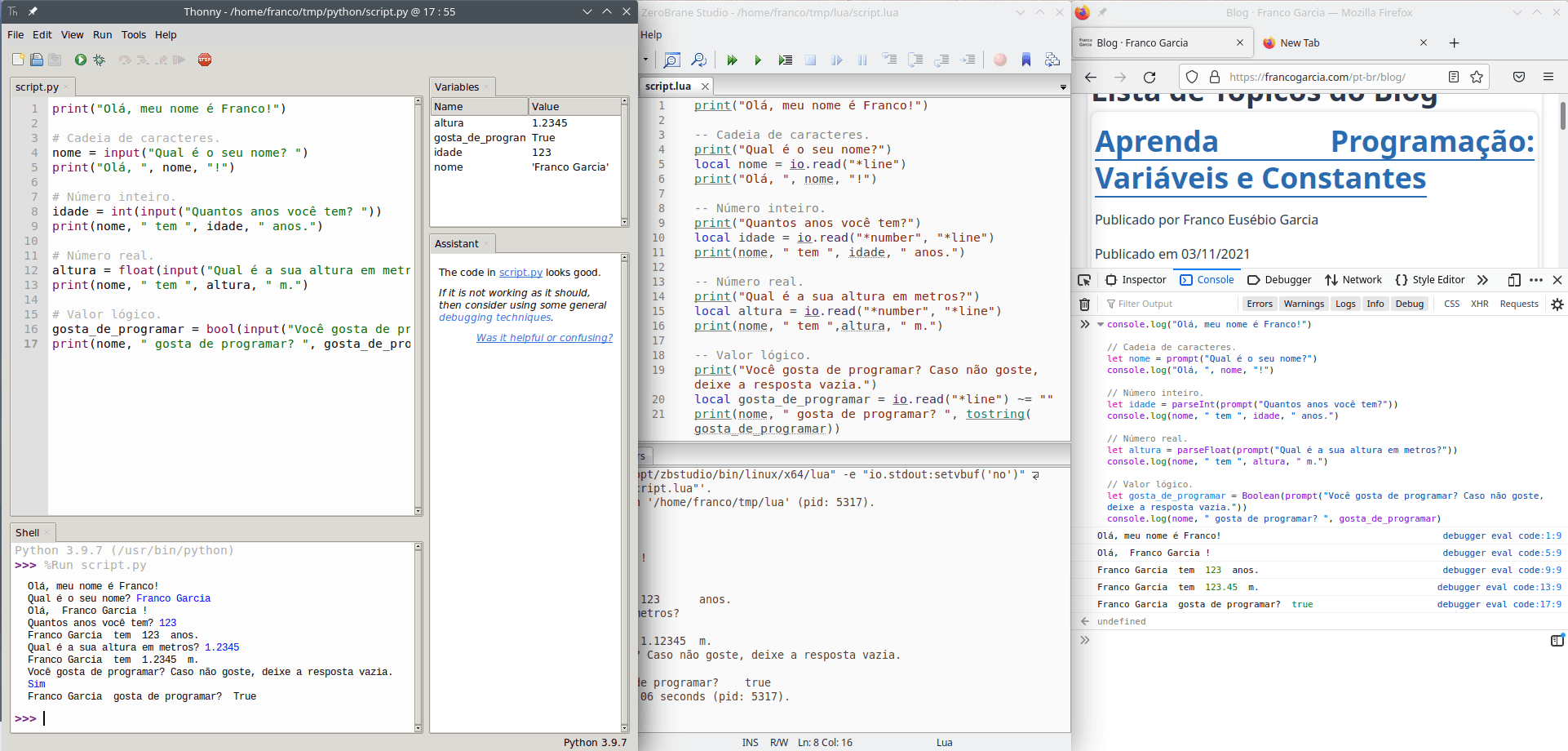
Image credits: Image created by the author using the program Spectacle.
Requirements
In the introduction to development environments, I have mentioned Python, Lua and JavaScript as good choices of programming languages for beginners. Later, I have commented about GDScript as an option for people who want to program digital games or simulations. For the introductory programming activities, you will need, at least, a development environment configured for one of the previous languages.
If you wish to try programming without configuring an environment, you can use of the online editors that I have created:
However, they do not provide all features offered by interpreters for the languages. Thus, sooner or later, you will need to set up a development environment. If you need to configure one, you can refer to the following resources.
Thus, if you have an Integrated Development Environment (IDE), or a combination of text editor and an interpreter, you are ready to start. The following example assumes that you know how to run code in your chosen language, as presented in the configuration pages.
If you want to use another language, the introduction provides links for configure development environments for the C, C++, Java, LISP, Prolog, and SQL (with SQLite) languages. In many languages, it suffices to follow the models from the experimentation section to modify syntax, commands and functions from the code blocks. C and C++ are exceptions, for they require pointers access the memory.
Programmers (or Developers) and End Users
Before starting your software development journey, your contact with computer programs was as a end user. End user is the person who uses a ready to use, implemented system. The term is the contrary of developer, which is the person that create systems.
Even as a programmer, you will always be an end user of other systems. For instance, except if you create every system in your computer (from the bootloader to the operating system, from the text editor to the compiler), you will use programs created by other people.
You have certainly created or inserted content in ready to use programs, without needing to modify them. For instance, you have accessed this page somehow. Perhaps you typed the address https://www.francogarcia.com in your browser, perhaps you used a search engine.
Whatever the path, you have, at some point, provided data to a system without writing source code. In this program, this happens through input. From this topic on, you will also be able to receive data provided by end users to process in your programs.
External Data and Hard Coded Data
Programs are composed of code and data. When you write the source code of a program, you create code as a combination of instructions and values. Values are data.
Without input, every data of a program must be defined in development time. This means that it is not possible to change a program without modifying the source code. To add or new data or to modify existing data, it is necessary to change the source code, recompile a run the program (or reinterpret it).
Input simplifies the process, because it allows using external data. The origin of external data is (as the name suggests) external to the program, which allows creating more flexible programs. Instead of predefined values, it is possible to obtain data from other sources, whenever necessary. In potential, the values can change at every use (instead of always being the same).
For instance, a calculator allows inputting numbers and operators to perform calculations. A calculator without input could only repeat predefined calculations written in the source code. You could create a calculator that always performed , though it would be very useful.
The same reasoning applies to any other interactive computer program. For instance, a browser without input would always show the same website. The page would be static, without any interactivity, because input are necessary to use links and scroll a page (to advance its contents). It would be possible to scroll content automatically, though it would be inconvenient. One possibility is defining a predefined processing (for instance, showing the next paragraph every 10 seconds). However, instead of an interactive browser, the result would be something like an informative or marketing panel.
In other words, input is fundamental for interactivity.
Hard Code
Data defined in the source code of a program are internal to it, and usually called hard coded. Unlike data obtained as input, it is only possible to change hard coded data by modifying the source code of the program.
Hard coded data are part of every program, because not every datum must be modified by end users. Every datum that is known during development time and that do not require changes when the program is used is a candidate to be hard coded. By analogy, every datum that is unknown during development time or that can change when the program is used is a strong candidate to become an input of the system.
More inputs result into greater system flexibility. Nevertheless, it also results into greater complexity of use. Therefore, for a good usability, it is prudent to balance input options with predefined behaviors. If behaviors must change, an alternative is providing default values. Thus, if a user wish to change them, she/he will have the option. Otherwise, she can use the system with greater convenience.
Code as Data or Data as Code
Some programming languages differ code created as instructions from code created as data in a program. Others do not, something that is called code as data (or data as code).
Programming languages that treat code as data allow the creation of extremely flexible and dynamic programs. This happens because, in these languages, end users can provide code as input as if they were data to modify parts of the program. In other words, end users can be programmers of the program being used.
This is possible because computer architectures normally follow the von Neumann architecture. The von Neumann architecture is also known as the stored program architectured, because it does not distinguish code from data in its memory. In other words, programs and data can co-exist in a same memory.
Of the most traditional examples of a language that allows using code as data is LISP. LISP, in particular, has a propriety called homoiconicity, that means that the language uses the same structure for code e data. In this case, s-expressions or sexps.
Code as data allow exploring advanced activities of metaprogramming. Although this is not a topic for this introduction, it is worth knowing the term, to know that is possible to write programs that generate other programs -- or even that allow the modification of the program being executed itself. The GNU Emacs text editor is a good example of a program that allows modifying itself when running.
Data Input
Data input usually is performed by reading strings. There are programming languages that allow reading formatted data, that is, that convert values from strings to other data types (usually primitive, such as integer numbers, real numbers and logic values). In the languages that do not permit it, the conversion must be performed manually, as previous detailed in data types. In this case, the conversion will occur from strings to the desired type.
Data Validation
In programs without input, all data is are defined by programmers. This means that, if a datum is incorrect, it was incorrectly defined by someone of the development team.
In programs with input, there is a new possibility for errors, caused by the provision of incorrect or unexpected data. If an end user provides a value considered as incorrect by the program, the code must be robust enough to handle it. Otherwise, the program will crash or will generate incorrect results; evidently, these are undesirable scenarios.
The handling of data is commonly called data validation. The implementation of data validation depends on programming resources that have not been defined yet, such as conditional and repetition structures.
Thus, at this time, the examples will only store data in variables. It is not yet possible to characterize data as invalid, neither avoiding crashes when the format of provided data does not correspond to the type of the variable that will store the value. Data validation, thus, will be presented at an appropriate time.
Flowcharts: Flowgorithm
To add a block for data input, right-click an arrow and choose the option Input.
Type the name of a variable (it must have been previously defined) that will receive the read value (for instance, name
) and confirm.
When the program is running, the input of a value will be requested when the execution reaches an input block.
Then, you will need to type a value (for instance, My Name
), followed by the enter
(⏎
) key.
If you prefer, you can also use the available button (Enter).
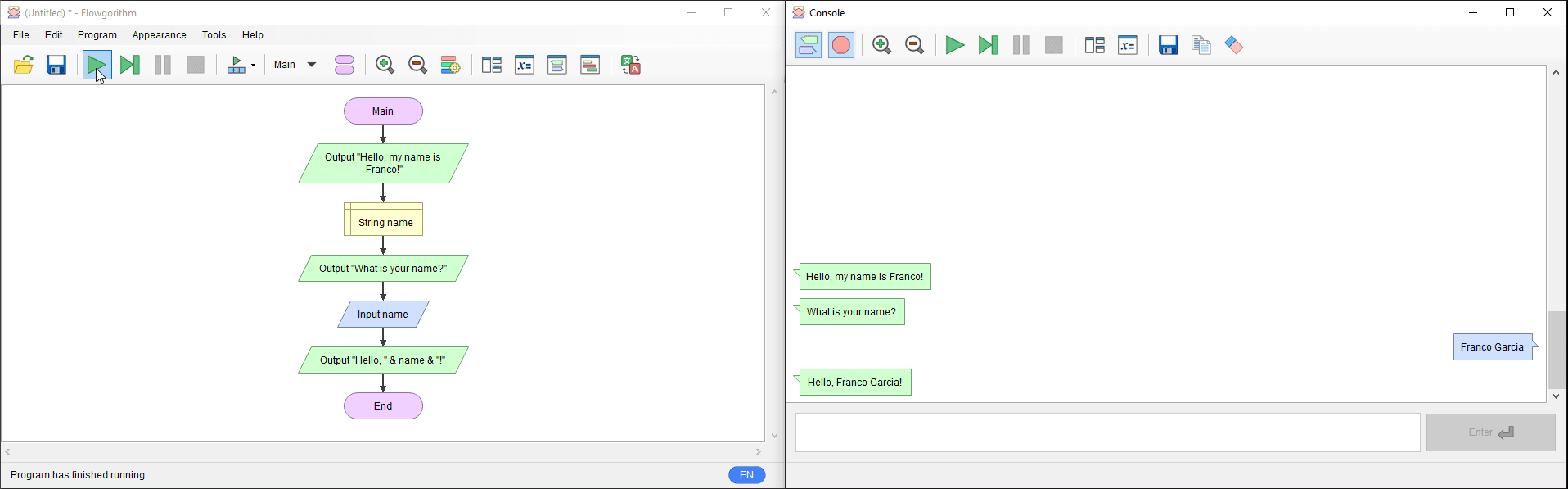
In the previous image, the flowchart has the following contents:
Main
Output "Hello, my name is Franco!"
String name
Output "What is your name?"
Input name
Output "Hello," & name & "!"
End
To write the value stored in the variable, you can use an output block.
If you wish to combine text with the value of the variable, you can write the desired text between double quotes, then write an ampersand (&
) followed by the name of the variable.
The process can be repeated as many times as required to write the message.
For instance, "Hello," & name & "!"
can write messages such as "Hello, my name is Franco!"
.
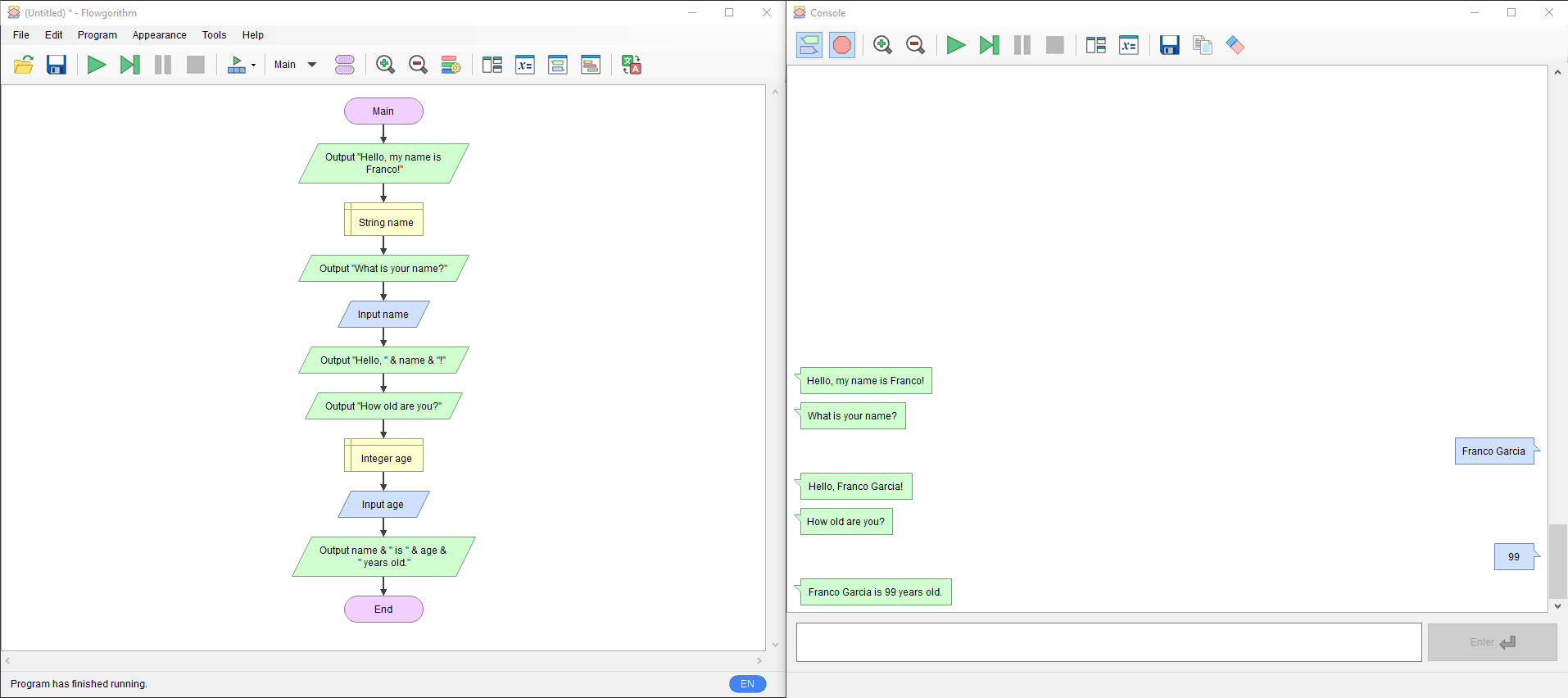
In the previous image, the flowchart has the following contents:
Main
Output "Hello, my name is Franco!"
String name
Output "What is your name?"
Input name
Output "Hello," & name & "!"
Output "How old are you?"
Integer age
Input age
Output name & " is " & age & " years old."
End
If the variables are of the logic type (Boolean
), the accepted values will be either true
or false
.
Other values are not allowed.
Visual Programming Language: Scratch
To add a block that reads data from the keyboard, access Sensing in the side panel, then choose _Ask What's your name?
and wait.
Adjust the message as necessary.
The answer will be stored in a variable called (answer), located after the input block.
To save the result, you can perform an assignment: copy the value of *answer to a variable of your preference (for instance, name
).
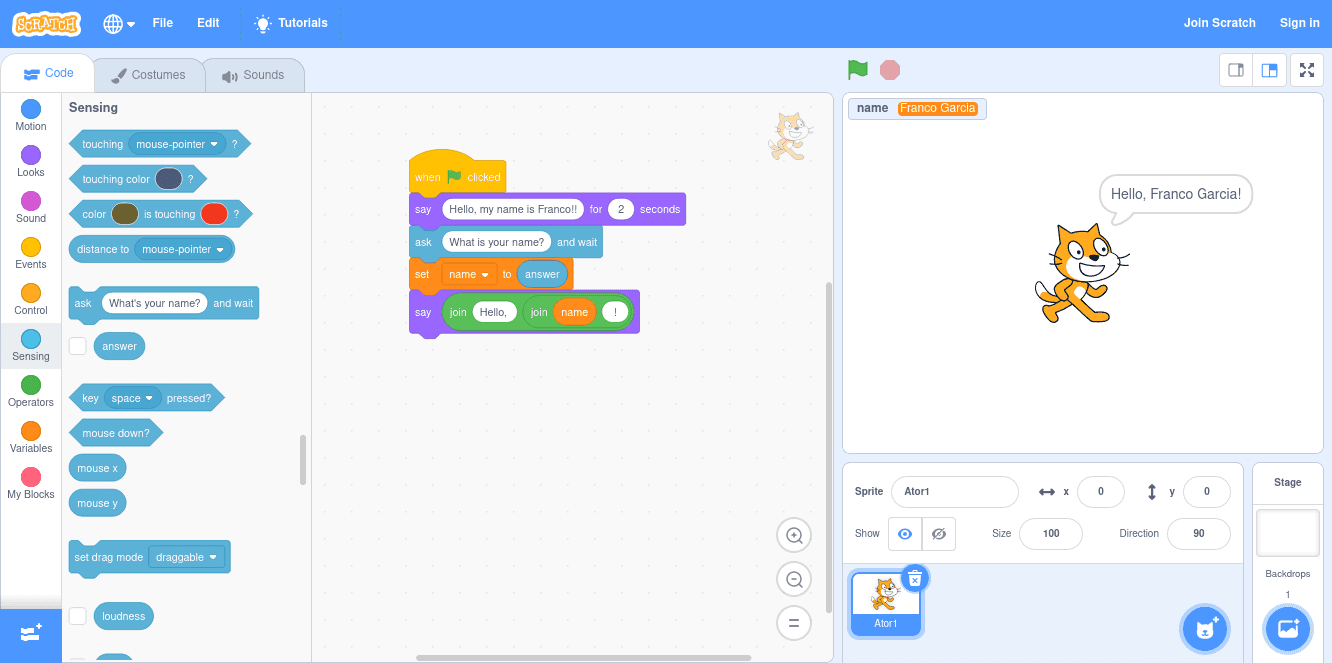
To write the value stored in the variable, you can use an output block.
If you wish to combine text with the value of the variable, access Operators, then choose the block Join apple
banana
.
Change the values by others of your preference.
To join several messages, it is possible to add the block Join apple
banana
inside other block (Join).
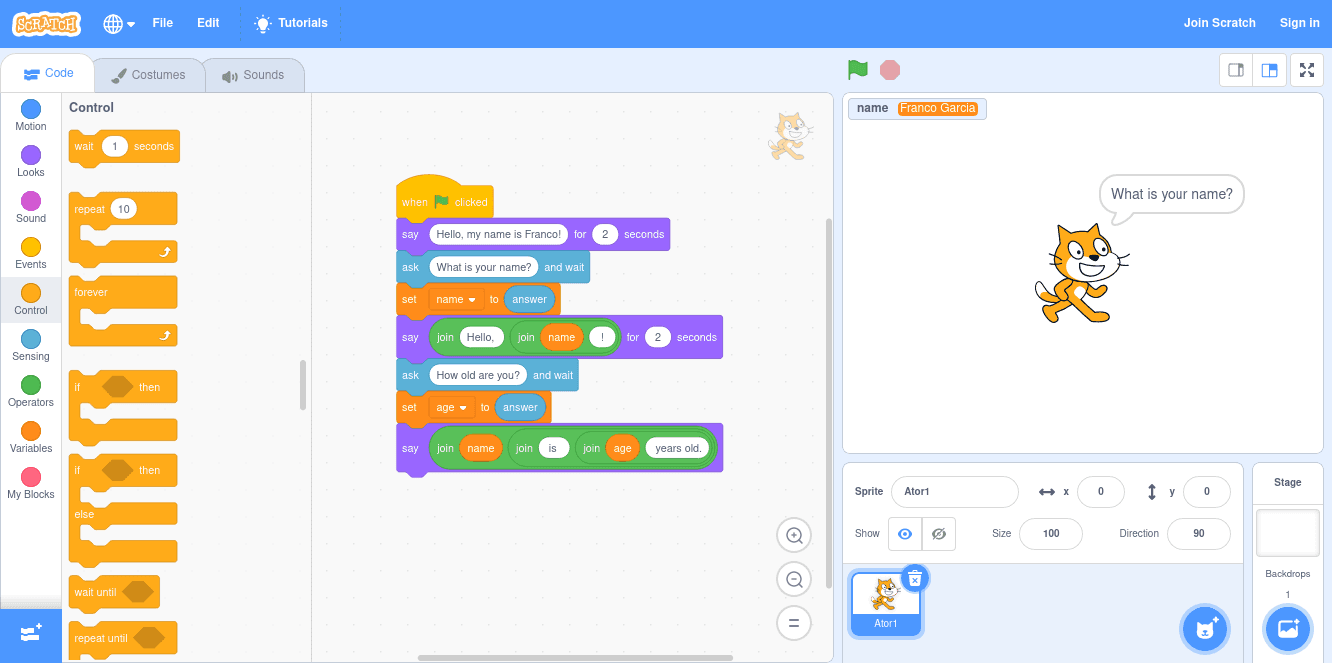
Textual Programming Language: JavaScript, Python, Lua and GDScript
It is simple to work with terminal (console) input in JavaScript, Python e Lua.
- In JavaScript, you can use
prompt()
: documentation. If you are using JavaScript in the command line, you can usereadline()
in SpiderMonkey or Node.js (documentation); - In Python, you can use
input()
: documentation. If you are using a REPL instead of a file with Python code, it is necessary to type (or copy and paste) and evaluate a line of code at a time. Otherwise, the request for input will be ignored by the interpreter. This problem does not happen in source code files; - In Lua, you can use
io.read()
: documentation.
In GDScript, it is not possible to read data from a terminal, as the engine presupposes the existence of a window.
Reading Strings
In general, the simplest way to request user input is to prompt the end user for a string. The reading occurs in a prompt.
console.log("Hello, my name is Franco!")
let name = prompt("What is your name?")
console.log("Hello, ", name, "!")
console.log("Hello, my name is Franco!")
console.log("What is your name?")
let name = readline()
console.log("Hello, ", name, "!")
print("Hello, my name is Franco!")
name = input("What is your name? ")
print("Hello, ", name, "!")
print("Hello, my name is Franco!")
print("What is your name?")
local name = io.read("*line")
print("Hello, ", name, "!")
The previous input is of the type blocking, which blocks the program execution until the person provides a value.
After the program writes What is your name?
, you should type the desired value, and, next, press enter
to sent it to the program.
For instance, My name
, followed by the enter
(⏎
) key.
In all previous examples, the values are stored in a variable called name
.
The value provided by the user can be accessed using the variable.
In Python and JavaScript (browser version), it is possible to modify the implementation as follows.
console.log("Hello, my name is Franco!")
console.log("What is your name?")
let name = prompt()
console.log("Hello, ", name, "!")
print("Hello, my name is Franco!")
print("What is your name?")
name = input()
print("Hello, ", name, "!")
In the modified version, the write command is used to show a message before requesting the value to be read. Thus, the resulting code resembles the one created for Lua and JavaScript (SpiderMonkey).
Reading Primitive Data Types
To complete the possible primitive type reading operations, the next code snippets illustrate how to read strings, integer numbers, real numbers and logic values from the console input.
console.log("Hello, my name is Franco!")
// String.
let name = prompt("What is your name?")
console.log("Hello, ", name, "!")
// Integer number.
let age = parseInt(prompt("How old are you?"))
console.log(name, " is ", age, " years old.")
// Real number.
let height = parseFloat(prompt("What is your height in meters?"))
console.log(name, " is ", height, " m.")
// Logic value.
let like_programming = Boolean(prompt("Do you like programming? If you do not, leave the answer empty."))
console.log("Does", name, " like to program? ", like_programming)
console.log("Hello, my name is Franco!")
// String.
console.log("What is your name?")
let name = readline()
console.log("Hello, ", name, "!")
// Integer number.
console.log("How old are you?")
let age = parseInt(readline())
console.log(name, " is ", age, " years old.")
// Real number.
console.log("What is your height in meters?")
let height = parseFloat(readline())
console.log(name, " is ", height, " m.")
// Logic value.
console.log("Do you like programming? If you do not, leave the answer empty.")
let like_programming = Boolean(readline())
console.log("Does", name, " like to program? ", like_programming)
print("Hello, my name is Franco!")
# String.
name = input("What is your name? ")
print("Hello, ", name, "!")
# Integer number.
age = int(input("How old are you? "))
print(name, " is ", age, " years old.")
# Real number.
height = float(input("What is your height in meters? "))
print(name, " is ", height, " m.")
# Logic value.
like_programming = bool(input("Do you like programming? If you do not, leave the answer empty. "))
print("Does", name, " like to program? ", like_programming)
print("Hello, my name is Franco!")
-- String.
print("What is your name?")
local name = io.read("*line")
print("Hello, ", name, "!")
-- Integer number.
print("How old are you?")
local age = io.read("*number", "*line")
print(name, " is ", age, " years old.")
-- Real number.
print("What is your height in meters?")
local height = io.read("*number", "*line")
print(name, " is ",height, " m.")
-- Logic value.
print("Do you like programming? If you do not, leave the answer empty.")
local like_programming = io.read("*line") ~= ""
print("Does", name, " like to program? ", like_programming)
In the previous snippets, it can be observed that Lua performs the appropriate conversion using a parameter. In the other languages, the conversion is performed manually.
Writing with Concatenation
In the output of some languages, the spacing between values can be irregular. To make it uniform, it is possible to create a string with the text to be written.
JavaScript converts values from primitive types to strings automatically. Lua does as well, except for logic values. In Python, the conversion must be performed manually.
console.log("Hello, my name is Franco!")
// String.
let name = prompt("What is your name?")
console.log("Hello, " + name + "!")
// Integer number.
let age = parseInt(prompt("How old are you?"))
console.log(name + " is " + age + " years old.")
// Real number.
let height = parseFloat(prompt("What is your height in meters?"))
console.log(name, " is " + height + " m.")
// Logic value.
let like_programming = Boolean(prompt("Do you like programming? If you do not, leave the answer empty."))
console.log("Does" + name + " like to program? " + like_programming)
console.log("Hello, my name is Franco!")
// String.
console.log("What is your name?")
let name = readline()
console.log("Hello + " + name, "!")
// Integer number.
console.log("How old are you?")
let age = parseInt(readline())
console.log(name + " is " + age + " years old.")
// Real number.
console.log("What is your height in meters?")
let height = parseFloat(readline())
console.log(name + " is " + height + " m.")
// Logic value.
console.log("Do you like programming? If you do not, leave the answer empty.")
let like_programming = Boolean(readline())
console.log("Does" + name + " like to program? " + like_programming)
print("Hello, my name is Franco!")
# String.
name = input("What is your name? ")
print("Hello, " + name + "!")
# Integer number.
age = int(input("How old are you? "))
print(name + " is " + str(age) + " years old.")
# Real number.
height = float(input("What is your height in meters? "))
print(name + " is " + str(height) + " m.")
# Logic value.
like_programming = bool(input("Do you like programming? If you do not, leave the answer empty. "))
print("Does" + name + " like to program? " + str(like_programming))
print("Hello, my name is Franco!")
-- String.
print("What is your name?")
local name = io.read("*line")
print("Hello, " .. name .. "!")
-- Integer number.
print("How old are you?")
local age = io.read("*number", "*line")
print(name .. " is " .. age .. " years old.")
-- Real number.
print("What is your height in meters?")
local height = io.read("*number", "*line")
print(name .. " is ".. height .. " m.")
-- Logic value.
print("Do you like programming? If you do not, leave the answer empty.")
local like_programming = io.read("*line") ~= ""
print("Does" .. name .. " like to program? " .. tostring(like_programming))
Terminal value input is simple. The previous examples are sufficient for learning activities.
Reading a String Can Fail in Lua (or Other Programming Languages, Such as C)
Nevertheless, there is a case that deserves attention. In Lua (and in some other programming languages, such as C), an attempt of reading a string after reading a number can fail.
print("i = ")
local i = io.read("*number")
print("i = ", i)
print("s = ")
local s = io.read("*line")
print("s = ", s)
In the previous example, a read for s
will not be requested.
s
will receive an empty value.
Some implementation for console read ignore certain values, such as line breaks provided after enter
is pressed to confirm the input of a value.
The ignored value persists for next reads (in an abstraction called stdin
).
However, a line break or an empty string are valid strings.
Consequently, the next attempt to read a value will fetch that value as input.
As a workaround to the problem, it is possible to repeat the reading of the string. The first attempt to read will retrieve the ignored character; the second will, effectively, request the input of a value.
print("i = ")
local i = io.read("*number")
print("i = ", i)
print("s = ")
local s = io.read("*line")
s = io.read("*line")
print("s = ", s)
It is also possible to request the input to ignore the superfluous value when reading a number. This is the approached use in the introductory example. If you thought it was strange that reading a number took two parameters, now you understand the reason for such use.
print("i = ")
local i = io.read("*number", "*line")
print("i = ", i)
print("s = ")
local s = io.read("*line")
print("s = ", s)
Both approaches are valid; you can choose the one you prefer.
GDScript
For GDScript input, it is necessary to create a graphical user interface with some elements to read and process the input. As the creation of programs with graphical user interface requires additional concepts, it will not be detailed in this page. The way to manipulate data is similar to the console's, though the required code is slightly more complex.
If you are curious (or wish to create a simple program with graphical interface), you can find an example in the page about configuration of development environment for GDScript (Godot). The description about how to proceed will be in the item about input.
If you opt to perform the steps, the result will be a program with a form to read data.
New Items for Your Inventory
Tools:
- Code as data.
Skills:
- Request for input of external data using console;
- Storage of data received as input in variables.
Concepts:
- External data;
- Von Neumann architecture;
- Input;
- Hard coded;
- Validation.
Programming resources:
- Data input.
Practice
If you have completed the practice proposed in Variables and Constants, you can continue to sophisticate your programs by requesting data input for each variable that you have created.
With variables and data input, it is possible to start writing your first customizable programs. For instance, you can create programs that generate messages such as cards.
Happy birthday, {NAME}!
Congratulations on becoming {AGE} years old!
May you have {WISH_1}, {WISH_2} and {WISH_3}!
You can also create exercises to fill the gaps (though it is still not possible to correct them automatically).
I like {PRIMEIRO_INTERESSE}.
I also like {SEGUNDO_INTERESSE}.
Coming to think, I prefer {SEGUNDO_INTERESSE} to {PRIMEIRO_INTERESSE}.
What about a model for preparing foods?
Ingredients:
- {INGREDIENT_1};
- {INGREDIENT_2}.
Preparation Mode:
Add {INGREDIENT_1} and {INGREDIENT_2} into a {container}.
Take it to an oven pre-heated to {TEMPERATURE}°C.
After {TIME} minutes, your recipe will be ready.
Perhaps a file for exercises?
Training Plan for {DAY}/{MONTH}/{YEAR}
{GOAL}
1. {EXERCISE_1}: {NUMBER_OF_SETS_1} x {NUMBER_OF_REPETITIONS_1}.
- Notes: {NOTES_1}
2. {EXERCISE_2}: {NUMBER_OF_SETS_2} x {NUMBER_OF_REPETITIONS_2}.
- Notes: {NOTES_2}
3. {EXERCISE_3}: {NUMBER_OF_SETS_3} x {NUMBER_OF_REPETITIONS_3}.
- Notes: {NOTES_3}
With variables and input, you can run each program many times to fill them with different values. By the way, can you observe the resemblance with forms in Web pages?
Name: {NAME}
Address:
Street: {STREET}
Number: {NUMBER}
Neighborhood: {NEIGHBORHOOD}
City: {CITY}
State: {STATE}
Postal Code: {POSTAL_CODE}
+--------+
| Submit |
+--------+
Start paying attention to the systems that you use. Identify inputs and imagine how the read data are processed. After all, you are on step way to start processing data in your own programs.
Next Steps
Systems have inputs, processing, and output. Now you know the beginning and end. It is time to learn how to implement the middle, to become able to process data from the input into answers as outputs.
- Introduction;
- Entry point and program structure;
- Output (for console or terminal);
- Data types;
- Variables and constants;
- Input (for console or terminal);
- Arithmetic and basic Mathematics;
- Relational operations and comparisons;
- Logic operations and Boolean Algebra;
- Conditional (or selection) structures;
- Subroutines: functions and procedures;
- Repetition structures (or loops);
- Arrays, collections and data structures;
- Records (structs);
- Files and serialization (marshalling);
- Libraries;
- Command line input;
- Bitwise operations;
- Tests and debugging.