Learn Programming: Entry Point (main()) and Program Structure
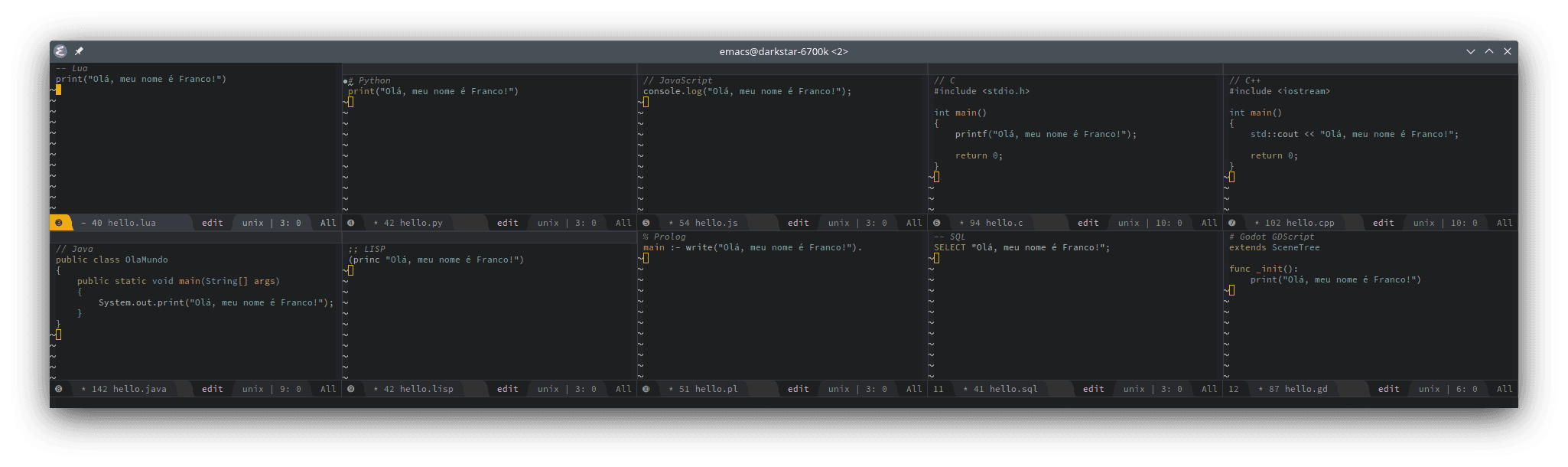
Image credits: Image created by the author using the program Spectacle.
Requirements
In the introduction to development environments, I have mentioned Python, Lua and JavaScript as good choices of programming languages for beginners. Later, I have commented about GDScript as an option for people who want to program digital games or simulations. For the introductory programming activities, you will need, at least, a development environment configured for one of the previous languages.
If you wish to try programming without configuring an environment, you can use of the online editors that I have created:
However, they do not provide all features offered by interpreters for the languages. Thus, sooner or later, you will need to set up a development environment. If you need to configure one, you can refer to the following resources.
Thus, if you have an Integrated Development Environment (IDE), or a combination of text editor and an interpreter, you are ready to start. The following example assumes that you know how to run code in your chosen language, as presented in the configuration pages.
If you want to use another language, the introduction provides links for configure development environments for the C, C++, Java, LISP, Prolog, and SQL (with SQLite) languages. In many languages, it suffices to follow the models from the experimentation section to modify syntax, commands and functions from the code blocks. C and C++ are exceptions, for they require pointers access the memory.
Every Program Has a Beginning
Your programming journey has a beginning. You are on your first steps.
Every program does also have a beginning. However, not all programs start from the first line defined in a source code file.
The beginning of a program vary according to the conventions established by the chosen programming language. Some languages consider that the first line with code in a source code file starts the program. Others define that every program must start from a reserved word, a command or a function.
Regardless of the case, the beginning of a program is called entry point.
Languages such as JavaScript and Lua define that the entry point is the first line of code from an interpreted file.
Languages such as C and Java use a function or method, called main()
, on which the initial code must be implemented.
Other languages can define different conventions to start a program.
Similarly, libraries, frameworks or engines can establish their own conventions regarding how a program implemented using them should start.
This is the case for _ready()
and _init()
in GDScript.
Structure of a Trivial Program
Regardless of case, source code written in a program language must follow the structure proposed by the language. It cannot be written anyhow, as is the case for natural languages. In a text, it is possible to write words at random, even if the combination does not make sense. In a programming language, choosing random words hardly ever results in a valid program. Very likely, the result will be an error emitted by the interpreter or the compiler.
Thus, one of the first steps to program in a language is to recognize the expected structure. Pattern recognition (or pattern matching) is an important skill for programming; it is time to practice it. The Section "Hello, World!" from the introduction to development environments show, in many languages, one of the simplest programs to create: a program that writes a message in the screen (usually of a console or terminal) and finishes.
For convenience, the examples are repeated in this section.
For each of the following languages, try to observe the structure of a program that writes Hello, my name is Franco!
.
What are the languages with similar syntax?
What are the languages with different syntax?
In your opinion, do some languages seem easier than others?
In Lua:
print("Hello, my name is Franco!")
In Python:
print("Hello, my name is Franco!")
In JavaScript:
Are you using a browser in a computer instead of a mobile device (smartphone or tablet)? If you are using a computer, you can run JavaScript code using the built-in console, accessible with the shortcut key
F12
.console.log("Hello, my name is Franco!")
In C:
#include <stdio.h> int main() { printf("Hello, my name is Franco!"); return 0; }
In C++:
#include <iostream> int main() { std::cout << "Hello, my name is Franco!"; return 0; }
In Java:
public class OlaMundo { public static void main(String[] args) { System.out.print("Hello, my name is Franco!"); } }
In LISP:
(princ "Hello, my name is Franco!")
In Prolog:
main :- write("Hello, my name is Franco!").
In SQL (SQLite):
SELECT "Hello, my name is Franco!";
In GDScript (Godot):
extends SceneTree func _init(): print("Hello, my name is Franco!")
Programs that start with a command or a function call to print are written in languages that assume that the first line of a file is the entry point of the program. The approach is common in interpreted languages, especially the ones that allow interactive programming using Read, Evaluate, Print, Loop (REPL). In the previous examples, Lua, Python, JavaScript, LISP, Prolog e SQL use the first line with code as the beginning of the program.
Programs that start with a command or a function such as main()
or _init()
are written using languages that define a specific entry point.
If you write the line with the instruction to print the message outside the command or function, it would result into an error.
C, C++, Java and GDScript are examples of languages with a specific entry point.
By default, none of the languages provide REPL features (though it is technically possible to implement one).
Some languages, like Python, optionally allow to define a specific entry point for a program.
For instance, the following Python source code does also write Hello, my name is Franco!
and finishes.
def main():
# [2] Then it comes here.
print("Hello, my name is Franco!")
if (__name__ == "__main__"):
main() # [1] The program starts here. # [3] It ends here.
Therefore, if you find a Python source code snippet that resembles the previous one, the program begins after the line started by the if
; in the example, that means it begins in line 6, not in line 1.
In fact, it starts in line 6, then executes line 3, then finishes at line 7.
In other words, the order that a computer follow the instructions it not, necessarily, the order on which the program was written. Likewise, not every line is executed in every use of a program. The reasons for the previous situations will become clear in the future, when concepts such as conditional structures, repetition structures, and subroutines (functions, procedures and methods) are introduced.
Basic Structure of Programs
A high-level structure for every computer program can be generalized as follows:
- Imports for libraries and predefined source code to aid the solution;
- Declarations for auxiliary code (for instance, data structures and subroutines);
- Main program:
- Entry point;
- Features of the main program;
- Exit point.
In the form of an algorithm, the structure can be represented as:
// Libraries and predefined code to help in the solution.
// Declaration for auxiliary auxiliary code (for instance, data structures and subroutines).
// Entry point.
begin
// Main program features.
// ...
// Exit point.
end
In the algorithm, the sequence started by two forward slashes //
are comments, that is, text that is ignored by a compiler or interpreter, and, thus, is not incorporated to a program.
Comments are written in natural language; they are not required to follow the syntax of the programming language.
Comments are useful to explain how the code works or the considered problem, for annotations, express ideas to others, and anything else that you consider that deserves to be written.
The takeaway is to know that is possible to add or remove comments without modifying how a program work.
For instance, both JavaScript source code snippets are equivalent:
Snippet without comments:
console.log("Hello, my name is Franco!")
Snippet with comment:
// Ignored line. // Another ignored line. // Now the program will start. console.log("Hello, my name is Franco!") // This is also ignored. // This line will also be ignored. // ...
Evidently, the previous comments are only illustrative. Irrelevant comments do not need to be written; they only add unnecessary content to the code. Although they do not make a difference for the machine, they do consume time and efforts from people reading the code.
To illustrate program structure for a real program, we can choose a language with a specific entry point. Code written in C provides a classical example of program structure.
// Libraries and predefined code to help in the solution.
#include <stdio.h>
// Entry point.
int main()
{
// Main program features.
printf("Hello, my name is Franco!");
// Exit point.
return 0;
}
The new code is equivalent to the one previously written in C, because, again, comments are ignored by the machine. Comments are for people, source code is for the machine (and for the people who read it).
As a curiosity, every program begins from a single entry point. However, there can exist multiple exit points. Programs can finish before the last instruction due to errors (called run-time errors), or in arbitrary points defined in the source code.
If you are wondering about the meaning of the 0
value in return 0
in the C code, it means success, that is, that the program finished correctly.
Beyond the Implementation
To stimulate your pattern recognition skills, can you imagine the reason why all programs written on this page are similar?
The programs that written Hello, my name is Franco!
are similar because all of them implement a very same algorithm.
In this case, a program that starts, write a predefined message, then ends.
It could be represented as:
begin
write('Hello, my name is Franco!')
end
In the previous algorithm, the program starts after begin
and finishes in end
.
The code written in between begin
and end
is the code of the program.
It is important to identify an algorithm because algorithms can be implemented in any programming language. To do so, it suffices to recognize the patterns.
In this case, to write the program Hello, world!
in an arbitrary programming language,
- You should know where the program starts. Is it in the first line of code of a file? Is it inside a specific code block?
- You should search for a command or subroutine (function, procedure or method) that can write a message in the terminal.
- You should know where a program ends.
For a practical example, let us choose a new programming language. For instance, C#.
The first step is try to discover what is the C# entry point. To do this, you can search for "C# entry point" in a search engine (for instance, DuckDuckGo, Google, or Bing). Another option is searching for "C# documentation entry point", to suggest to the search engine that it should find entries with the keyword "documentation". Next, you could search for "C# print", to try to discover what is the resource to print to the console in C#. With the previous information, you could write your first program in C#.
Now that you know the program Hello, World!
, a third option is searching for "C# hello world".
Hello, World!
allows you to quickly identify the expected structure for a program in a programming language.
In other words, Hello, World!
is a great choice to start learning a new programming language.
Regardless of path, the search should reveal that a C# program has the following structure:
using System;
class MyClass
{
static void Main(string[] args)
{
Console.WriteLine("Hello, my name is Franco!");
}
}
The C# program is, thus, similar to one written in languages such as C, C++ and Java. By the way, the C# program is very similar to the Java one.
The next step to start programming activities in C# is finding how to run source code written in the language (you can search for something like "c# run program documentation"). However, as it is not the goal for this topic, we can continue.
C# is a popular programming language. For a second example, we can consider the esoteric programming language LOLCODE. An esoteric programming language (or esolang) is commonly created as a joke.
HAI 1.2
CAN HAS STDIO?
VISIBLE "Hello, my name is Franco!"
KTHXBYE
If one follows the same steps, the results could be a program such as the previous one. Can you identify the basic structure of a program in LOLCODE?
To learn more about search engines, search for how to make advanced searches. For instance, double quotes allow searching for exact terms; it is also worth knowing how to filter results by date (especially year, in the case of programming, to find more up-to-date results). Thus, consult the documentation of your favorite search engine; for instance, for Google (also check this). For DuckDuckGo, it is worth learning about bangs.
Computational Thinking in Action
The three steps to write a Hello, World!
program in a new programming language provide an example of applying computational thinking.
The original problem was to write Hello, World!
in a new programming language -- in this case, C# and LOLCODE.
Next, we decomposed the problem in smaller parts: identify the entry point, identify the required resource to write a message, identify how to finish the program.
- Identify the entry point;
- Identify the required resource to write a message;
- Identify how to finish the program;
- Create a new text file;
- Write, if necessary, the library imports in the file;
- Write the entry point to the file;
- Write the required code to write the message (and the message) in the file;
- Write the exit point to the file.
Although the level of the previous algorithm is too high for computers, it is valid for people. In other words, a computer and a program language are necessary, though insufficient to program. Programming is, above all, an intellectual practice on which you solve problems in a structured and repeatable way. For computer programming, you must convert a solution expressed in human thought in steps that are small and simple enough to be repeated by a machine.
Therefore, programming is not memorizing the syntax of a programming language. You can refer to the syntax of a language whenever required. To do this, it suffices to search the documentation.
Programming is solving problems and demonstrating to a machine (the computer) how to repeat the conceived solution. The computer is a machine without intelligence (that is, an unintelligent machine), thus the conceived solution cannot afford mistakes, nor ambiguities (different possibilities for interpretation). The steps must be compatible with the available instructions provided by the computer architecture, which are what the computer can do.
What do you do when you know what to do, thought now how to do it? You study, research and use a search engine.
Search engines are one of the most useful tools for programmers. However, you must know what to search. If you know what you need, you can find how to do it.
To know what you need, you must understand the fundamentals. Fundamentals are related to the concepts and basic instructions that a computer can perform.
The next topics describe some fundamentals. Before proceeding, it is convenient to organize the way of thinking, for research and study are essential to program well.
Scientific Method
Part of thinking computationally is thinking like a scientist. The scientific method is, thus, applicable for programming. From here on, it should also be part of your tools.
Simplify (and informally), the scientific methods consist of:
- Identify a problem;
- Research about what is known about the problem;
- Formulate hypothesis;
- Plan experiments;
- Test and evaluate the obtained results;
- Describe results, draw conclusions, and reflect about what you learned;
- Repeat until the problem is solved (or you are satisfied with the outcome).
If you wish, you can change scientist to detective. The method is still valid.
For the example of Hello, World!
in C#, the method scientific was applied as:
- Problem characterization: a C# program that writes
Hello, World
in the console; - Formulate hypothesis: with a resource to write in the console placed in between the entry and exit point, a program to write a message in the console could be written;
- Plan experiments:
- Search for the required elements for the premise of the hypothesis: entry point, exit point, write resource;
- Write a source code with the identified elements;
- Run the created program;
- Compare the results.
If the output was
Hello, my name is Franco!
, then the conclusion (thesis) is correct: the three elements allow creating a program to write a message in the console; Otherwise, analyze the results, try to better understand the problem, reformulate the hypothesis, repeat the process.
- Test and evaluate the results;
- Your conclusions.
Strictly speaking, a scientific process requires greater methodological rigour and derailment. However, as it is not an academic problem, the most important is understanding the process and how to think to find solutions for problems.
In sum, the better your understanding of your problem, the clearer and simpler will be the solution. Clear and simple solutions tend to be elegant ones; long for elegance.
Scientific Method and Entry Point
What is the relation of the scientific method with an entry point of a program?
None. Every.
Algorithms are finite sequence of steps to solve problems. If there is not a goal, there is not an end. Is there does not exist an end, there does not exist an algorithm. In other words, an algorithm must end.
Every program must transition from an initial state and reach a desired final state. Every instruction of a code try to approximate the current state to the final state.
If an instruction does not contribute with the solution, question whether it should appear in your source code.
Not Every Valid Program is Correct
The title of the subsection seems like an oxymoron, thought it is correct.
Compilers and interpreters can detect syntax errors in the use of a language, thought they cannot inform whether a program is correct (the reason is the halting problem, that will be cited in the next subsection). A source code with correct syntax, but incorrect results (or behaviors), is called with logic error.
In other words, a program valid in a program logic is not, necessarily, a correct program. Thus, the work of a developer does not end at the moment that the program is running. Correct results require performing inspections and tests. It is important to define expected results from given inputs to verify if a program seems to be correct. That is, the scientific method proves to be useful once again, for hypothesis tests and validation.
Not Every Program Ends
There are problems that demand such large quantities of time that they are unsolvable with the current technology. It is possible to approximate them, thought it is not possible to solve them. The study of the complexity of problems is called computational complexity.
In simple terms, this implies that there exists problems that cannot be solved with computers. Thus, for practical purposes, computationally possible problems and beginner programs, there are two types of programs that do not end:
- Incorrect programs;
- Programs with an algorithm that (purposely) is repeated forever.
In other words, if you write a program that, inadvertently, does not end, there probably exists a logic error in your project.
The reason for the choice of the term "probably" instead of "certainly" is that there exists problems that truly are impossible to solve with current computers. One is called the halting problem, to determine whether, given a program specification and an input (whatever it is), the program will end.
The halting problem is the reason why an interpreter or a compiler cannot affirm if any program that can be written is correct. For a counterexample, you can imagine the case of programs that repeat forever, that is, that intentionally should not end. To allow the interpreter or compiler to affirm whether such program is correct, the program must end. In other words, the program would have, simultaneously, to end and never to end. If this seems to be confusing and impossible, it is because it truly is a contradiction.
The takeaway is to know that, for complex problems, it can be hard to prove whether an algorithm is correct -- or even if it will finish. This is relevant because there are systems on which any mistake can be fatal, such as systems for healthcare or transportation.
New Items for Your Inventory
Tools:
- Programming language;
- Search engine;
- Scientific method.
Skills:
- Pattern recognition (pattern matching).
Concepts:
- Program structure;
- Programs that finish (or not).
Programming resources:
- Comments.
Next Steps
Although the interactivity of this page is almost null, the content is of great importance. One of the most important programming skills is the capacity of solving problems. In this page, you have started to think as a programmer to solve problems. Hereafter, apply the scientific method and the decomposition from the computational thinking every time you program.
You have learned how to structure programs in programming languages. Not only in some, though in any language. After all, now you know how to proceed whenever you want to learn a new language.
In fact, this shows the power of the computational thinking. Learn one programming language, and you will know the chosen programming language. Learn computational thinking, and you will be able to program in any programming language.
You have also learned how to use search engine as tools to assist programming. The ability to search effectively to find the desired answers is an important skill for software development.
Furthermore, you have learned to identify where the implementation starts in a file, as well as where it should finish. Your programming journey has also started here. You are the only one who can determine when it will end. The more you study, learn, practice and create, the better programmer you will become.
- Introduction;
- Entry point and program structure;
- Output (for console or terminal);
- Data types;
- Variables and constants;
- Input (for console or terminal);
- Arithmetic and basic Mathematics;
- Relational operations and comparisons;
- Logic operations and Boolean Algebra;
- Conditional (or selection) structures;
- Subroutines: functions and procedures;
- Repetition structures (or loops);
- Arrays, collections and data structures;
- Records (structs);
- Files and serialization (marshalling);
- Libraries;
- Command line input;
- Bitwise operations;
- Tests and debugging.